As you know, we can perform CRUD operations in MVC 5 with an Entity Framework Model and we also work in Paging & Searching in MVC 5. Now, suppose we want to update the database with new details in the Students, Courses and Enrollments entities, then we need to migrate our application.
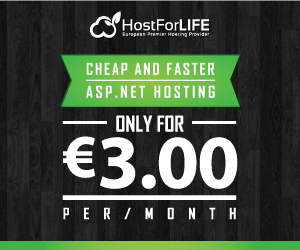
In that context, you'll learn today to do the Code First Migration in the MVC application. The migration helps you to update and change the data model that you've created for the application and you don't need to re-create or drop your database. So, let's proceed with the following section.
Apply Code First Migration
The data model in the database usually changes after developing the new application. When the data model changes, it becomes out of sync with the database. We've configured our Entity Framework in the app to drop and recreate the data model automatically. When any type of change is done in the entity classes or in the DbContext class, the existing database is deleted and the new database is created that matches the model and sees it with test data.
When the application runs in production, we do not want to loose everything each time we make changes like adding a column. The Code First Migration solved this problem by enabling the code first to update the database instead of dropping or recreating.
Use the following procedure to create a sample of that.
Step 1: Please disable the Database Initializer in the Web.Config file or in the Global.asax file like as in the following:
In Web.Config File:
<!--<contexts>
<context type="Vag_Infotech.DAL.CollegeDbContext, Vag_Infotech">
<databaseInitializer type="Vag_Infotech.DAL.CollegeDatabaseInitializer, Vag_Infotech"></databaseInitializer>
</context>
</contexts>-->
In Global.asax File:
// Database.SetInitializer(new CollegeDatabaseInitializer());
Step 2: Please change the database name in the Web.Config file like as in the following:
<connectionStrings>
<add name="CollegeDbContext" connectionString="Data Source=(LocalDb)\v11.0;Initial Catalog=VInfotechNew;Integrated Security=SSPI" providerName="System.Data.SqlClient" />
</connectionStrings>
Step 3: Open the Package Manager Console and enter the following commands:
enable-migration
add-migration InitialCreate
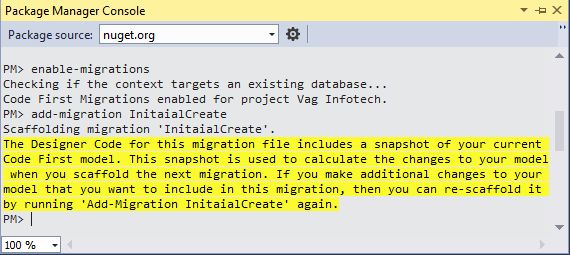
It'll create the Migration folder in your application and Configuration.cs file in it automatically.
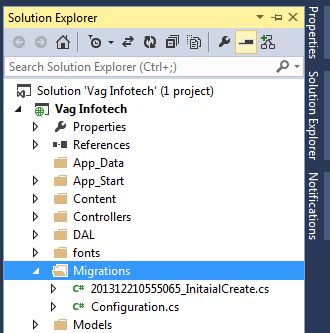
You can see the Seed() created in the file and the purpose of this method is to enable the insert or change data after Code First creates or updates the database.
Editing Seed Method
Now, we add new code for updating our data model in this method. When we do not use the migration, the data model is dropped or re-created during the execution of the application each time, but now the data is retained after database changes.
Step 1: Modify your Configuration.cs with the following code:
namespace Vag_Infotech.Migrations
{
using System;
using System.Collections.Generic;
using System.Data.Entity;
using System.Data.Entity.Migrations;
using System.Linq;
using Vag_Infotech.Models;
internal sealed class Configuration : DbMigrationsConfiguration<Vag_Infotech.DAL.CollegeDbContext>
{
public Configuration()
{
AutomaticMigrationsEnabled = false;
}
protected override void Seed(Vag_Infotech.DAL.CollegeDbContext context)
{
var New_Students = new List<Student>
{
new Student{FirstName="Alec",LastName="Michael",
EnrollmentDate=DateTime.Parse("2009-07-01")},
new Student{FirstName="Marie",LastName="Jane",
EnrollmentDate=DateTime.Parse("2009-07-01")},
new Student{FirstName="Peter",LastName="Parker",
EnrollmentDate=DateTime.Parse("2009-07-05")},
new Student{FirstName="Tony",LastName="Stark",
EnrollmentDate=DateTime.Parse("2009-10-01")},
new Student{FirstName="Steve",LastName="Rogers",
EnrollmentDate=DateTime.Parse("2010-07-01")},
new Student{FirstName="Natasha",LastName="Romanoff",
EnrollmentDate=DateTime.Parse("2010-07-10")},
new Student{FirstName="Bruce",LastName="Banner",
EnrollmentDate=DateTime.Parse("2009-09-01")},
new Student{FirstName="Scott",LastName="Lang",
EnrollmentDate=DateTime.Parse("2009-08-05")},
};
New_Students.ForEach(ns => context.Students.AddOrUpdate(p => p.LastName, ns));
context.SaveChanges();
var New_Courses = new List<Course>
{
new Course{CourseID=201,Name="MCA",Credit=3,},
new Course{CourseID=202,Name="M.Sc.IT",Credit=2,},
new Course{CourseID=203,Name="M.Tech.CS",Credit=2,},
new Course{CourseID=204,Name="M.Tech.IT",Credit=4,}
};
New_Courses.ForEach(ns => context.Courses.AddOrUpdate(p => p.Name, ns));
context.SaveChanges();
var New_Enrollments = new List<Enrollment>
{
new Enrollment{
StudentID= New_Students.Single(ns=>ns.FirstName=="Alec").ID,
CourseID=New_Courses.Single(nc=>nc.Name=="MCA").CourseID,
Grade=StudentGrade.A
},
new Enrollment{
StudentID= New_Students.Single(ns=>ns.FirstName=="Marie").ID,
CourseID=New_Courses.Single(nc=>nc.Name=="MCA").CourseID,
Grade=StudentGrade.A
},
new Enrollment{
StudentID= New_Students.Single(ns=>ns.FirstName=="Marie").ID,
CourseID=New_Courses.Single(nc=>nc.Name=="M.Tech.IT").CourseID,
Grade=StudentGrade.B
},
new Enrollment{
StudentID= New_Students.Single(ns=>ns.FirstName=="Peter").ID,
CourseID=New_Courses.Single(nc=>nc.Name=="M.Sc.IT").CourseID,
Grade=StudentGrade.A
},
new Enrollment{
StudentID= New_Students.Single(ns=>ns.FirstName=="Tony").ID,
CourseID=New_Courses.Single(nc=>nc.Name=="MCA").CourseID,
Grade=StudentGrade.A
},
new Enrollment{
StudentID= New_Students.Single(ns=>ns.FirstName=="Tony").ID,
CourseID=New_Courses.Single(nc=>nc.Name=="M.Sc.IT").CourseID,
Grade=StudentGrade.B
},
new Enrollment{
StudentID= New_Students.Single(ns=>ns.FirstName=="Steve").ID,
CourseID=New_Courses.Single(nc=>nc.Name=="M.Tech.IT").CourseID,
Grade=StudentGrade.A
},
new Enrollment{
StudentID= New_Students.Single(ns=>ns.FirstName=="Natasha").ID,
CourseID=New_Courses.Single(nc=>nc.Name=="MCA").CourseID,
Grade=StudentGrade.B
},
new Enrollment{
StudentID= New_Students.Single(ns=>ns.FirstName=="Bruce").ID,
CourseID=New_Courses.Single(nc=>nc.Name=="M.Tech.IT").CourseID,
Grade=StudentGrade.B
},
new Enrollment{
StudentID= New_Students.Single(ns=>ns.FirstName=="Scott").ID,
CourseID=New_Courses.Single(nc=>nc.Name=="MCA").CourseID,
Grade=StudentGrade.A
},
new Enrollment{
StudentID= New_Students.Single(ns=>ns.FirstName=="Scott").ID,
CourseID=New_Courses.Single(nc=>nc.Name=="M.Tech.IT").CourseID,
Grade=StudentGrade.D
},
};
foreach (Enrollment et in New_Enrollments)
{
var NewEnrollDb = context.Enrollments.Where(
ns => ns.Student.ID == et.StudentID &&
ns.Course.CourseID == et.CourseID).SingleOrDefault();
if (NewEnrollDb == null)
{
context.Enrollments.Add(et);
}
}
context.SaveChanges();
}
}
}
In the code above the Seed() takes the context object as an input argument and in the code the object is used to add the entities to the database. We can see how the code creates the collection for the database for each entity type and then saves it by SaveChanges() and adds it through the DbSet property. It is not necessary to call the SaveChanges() after each group of entities.
Step 2: Build the solution.
Migration Executing
Step 1: Enter the following command in the Package Manager Console:
update-database
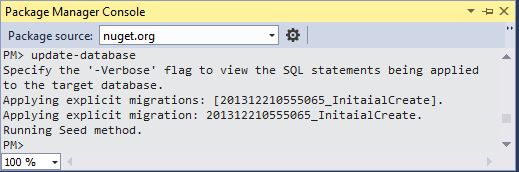
Step 2: Run the application and see the changes in the Students link.