In this article, I will explain how to generate 'Access Token' using credentials of 'Asp.net Identity' in 'ASP.Net MVC. Create a new project in Visual Studio.
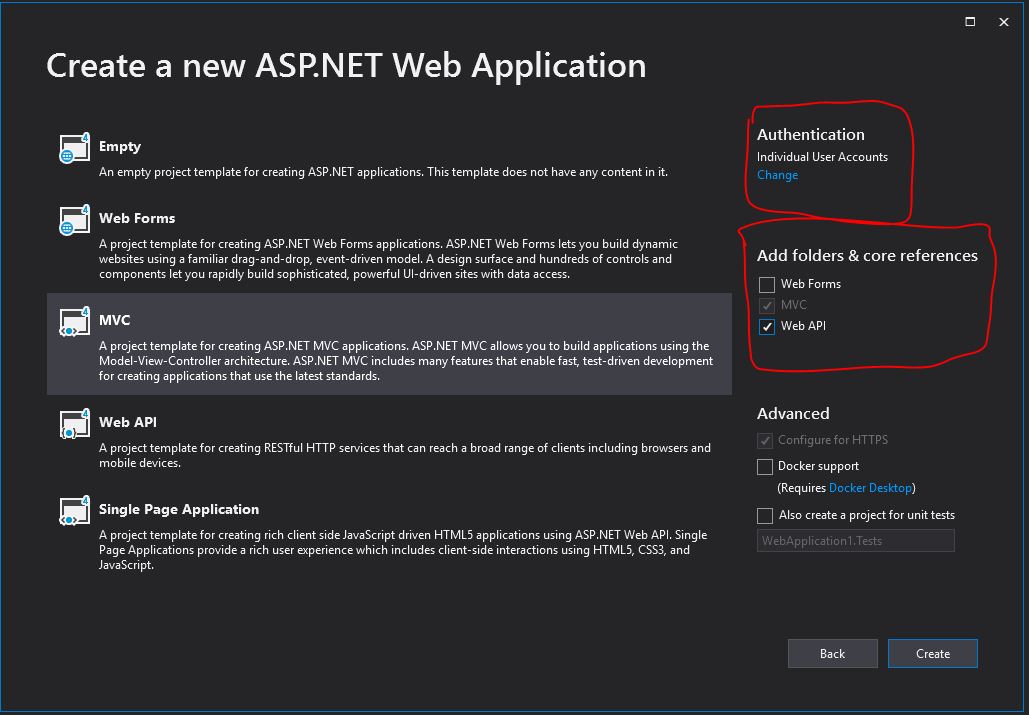
Give connection string of your database. Register an Account.
Add the following three Nuget Packages to your project.
- Microsoft.Owin.Host.SystemWeb
- Microsoft.Owin.Security.OAuth
- Microsoft.Owin.Cors
Now, add TokenGenerating.cs class in the project.
using Microsoft.AspNet.Identity;
using Microsoft.AspNet.Identity.EntityFramework;
using Microsoft.Owin.Security.OAuth;
using System;
using System.Collections.Generic;
using System.Linq;
using System.Security.Claims;
using System.Security.Cryptography;
using System.Threading.Tasks;
using System.Web;
namespace SecureWebAPI.APIClasses
{
public class TokenGenerating : OAuthAuthorizationServerProvider
{
public override async Task ValidateClientAuthentication(OAuthValidateClientAuthenticationContext context)
{
context.Validated(); //
}
public override async Task GrantResourceOwnerCredentials(OAuthGrantResourceOwnerCredentialsContext context)
{
UserManager<IdentityUser> userManager = new UserManager<IdentityUser>(new UserStore<IdentityUser>());
var result = userManager.Find(context.UserName, context.Password);
//UserManager<IdentityUser> userManager = new UserManager<IdentityUser>(new UserStore<IdentityUser>());
//var result = userManager.Find(context.UserName, context.Password);
//UserManager holds data for register user.
//context.UserName = Email of your registered user
//context.Password = Password of your registered user
if (result != null)
{
var identity = new ClaimsIdentity(context.Options.AuthenticationType);
context.Validated(identity);
}
else
{
context.SetError("invalid_grant", "Provided username and password is incorrect");
return;
}
}
}
}
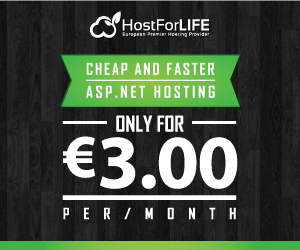
Now add a new startup class for the token configuration file this class holds the information and setting of the token.
using Microsoft.Owin;
using Microsoft.Owin.Security.OAuth;
using Owin;
using System;
using System.Threading.Tasks;
using System.Web.Http;
[assembly: OwinStartup(typeof(SecureWebAPI.APIClasses.AuthenticationStartupClass))]
namespace SecureWebAPI.APIClasses
{
public class AuthenticationStartupClass
{
public void Configuration(IAppBuilder app)
{
// For more information on how to configure your application, visit https://go.microsoft.com/fwlink/?LinkID=316888
app.UseCors(Microsoft.Owin.Cors.CorsOptions.AllowAll);
var myProvider = new APIAUTHORIZATIONSERVERPROVIDER();
OAuthAuthorizationServerOptions options = new OAuthAuthorizationServerOptions
{
AllowInsecureHttp = true,
TokenEndpointPath = new PathString("/token"),
AccessTokenExpireTimeSpan = TimeSpan.FromDays(1),
Provider = myProvider
};
app.UseOAuthAuthorizationServer(options);
app.UseOAuthBearerAuthentication(new OAuthBearerAuthenticationOptions());
HttpConfiguration config = new HttpConfiguration();
WebApiConfig.Register(config);
}
}
}
Add new class for API Attributes
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
namespace SecureWebAPI.APIClasses
{
public class APIAUTHORIZEATTRIBUTE : System.Web.Http.AuthorizeAttribute
{
protected override void HandleUnauthorizedRequest(System.Web.Http.Controllers.HttpActionContext actionContext)
{
if (!HttpContext.Current.User.Identity.IsAuthenticated)
{
base.HandleUnauthorizedRequest(actionContext);
}
else
{
actionContext.Response = new System.Net.Http.HttpResponseMessage(System.Net.HttpStatusCode.Forbidden);
}
}
}
}
Change Global.asax file of your project.
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Web.Http;
using System.Web.Mvc;
using System.Web.Optimization;
using System.Web.Routing;
namespace SecureWebAPI
{
public class MvcApplication : System.Web.HttpApplication
{
protected void Application_Start()
{
AreaRegistration.RegisterAllAreas();
WebApiConfig.Register(GlobalConfiguration.Configuration);
FilterConfig.RegisterGlobalFilters(GlobalFilters.Filters);
RouteConfig.RegisterRoutes(RouteTable.Routes);
BundleConfig.RegisterBundles(BundleTable.Bundles);
GlobalConfiguration.Configuration.EnsureInitialized();
}
}
}
Now change your WebApiConfig.cs file routemap
Your Project > App_Start folder > WebApiConfig.cs
routeTemplate: "api/{controller}/{action}/{id}",
using System;
using System.Collections.Generic;
using System.Linq;
using System.Net;
using System.Net.Http;
using System.Web.Http;
namespace SecureWebAPI.Controllers
{
public class UserController : ApiController
{
[AllowAnonymous]
[HttpGet]
public IHttpActionResult Get()
{
return Ok("Now server time is: " + DateTime.Now.ToString());
}
[Authorize]
[HttpGet]
public IHttpActionResult GetForAuthenticate()
{
return Ok("Hello ");
}
[Authorize]
[HttpGet]
public IHttpActionResult GetForAdmin()
{
return Ok("Helo User");
}
}
}
Add a ApiController .
using System;
using System.Collections.Generic;
using System.Linq;
using System.Net;
using System.Net.Http;
using System.Web.Http;
namespace SecureWebAPI.Controllers
{
public class UserController : ApiController
{
[AllowAnonymous]
[HttpGet]
public IHttpActionResult Get()
{
return Ok("Now server time is: " + DateTime.Now.ToString());
}
[Authorize]
[HttpGet]
public IHttpActionResult GetForAuthenticate()
{
return Ok("Hello ");
}
[Authorize]
[HttpGet]
public IHttpActionResult GetForAdmin()
{
return Ok("Helo User");
}
}
}
Run your Project and leave it. Open Visual Studio, add a new console project. Add a new class to the console project.
class TokenInfo
{
public string access_token { get; set; }
public string token_type { get; set; }
public int expires_in { get; set; }
}
Add function in Program.cs class.
public string GetAccessToken(string Email, string Password)
{
string AccessToken = "";
string responseFromServer = "";
WebRequest request = WebRequest.Create("https://localhost:44370/token"); //your project url
request.Method = "POST";
string postData = "username=" + Email + "&password=" + Password + "&grant_type=password";
byte[] byteArray = Encoding.UTF8.GetBytes(postData);
request.ContentType = "application/x-www-form-urlencoded";
request.ContentLength = byteArray.Length;
System.IO.Stream dataStream = request.GetRequestStream();
dataStream.Write(byteArray, 0, byteArray.Length);
dataStream.Close();
WebResponse response = request.GetResponse();
Console.WriteLine(((HttpWebResponse)response).StatusDescription);
using (dataStream = response.GetResponseStream())
{
System.IO.StreamReader reader = new System.IO.StreamReader(dataStream);
responseFromServer = reader.ReadToEnd();
Console.WriteLine(responseFromServer);
}
TokenInfo myDeserializedClass = Newtonsoft.Json.JsonConvert.DeserializeObject<TokenInfo>(responseFromServer);
AccessToken = myDeserializedClass.access_token;
response.Close();
return AccessToken;
}
MainMethod
static void Main(string[] args)
{
string Email = "Your Registered user Email";
string Password = "Your Registered user Email";
Program cls = new Program();
string AccessToken = cls.GetAccessToken(Email, Password);
HttpWebRequest request = (HttpWebRequest)WebRequest.Create("https://localhost:44370/api/user/GetForAuthenticate"); //Your project Local host api url
request.AutomaticDecompression = DecompressionMethods.GZip;
request.Method = "GET";
request.Headers.Add("Authorization", "Bearer " + AccessToken);
using (System.Net.WebResponse GetResponse = request.GetResponse())
{
using (System.IO.StreamReader streamReader = new System.IO.StreamReader(GetResponse.GetResponseStream()))
{
dynamic jsonResponseText = streamReader.ReadToEnd();
}
}
Console.ReadLine();
}
Run console project
If Credential is authenticated then an access token will also be generated.
Keep in mind Your Asp.net MVC project should be running during access token generating.