One year back I wrote the following article: Creating AutoComplete Extender using ASP.NET which has huge response. Currently it has 32 k views. So by considering same requirement in ASP.NET MVC I decided to write same type of article using ASP.NET MVC with the help of jQuery UI library. So let us implement this requirement step by step,
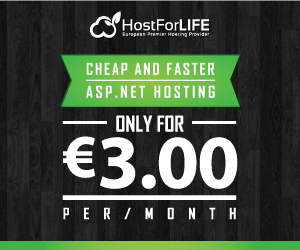
Step 1 - Create an ASP.NET MVC Application.
"Start", then "All Programs" and select "Microsoft Visual Studio 2015".
"File", then "New" and click "Project", then select "ASP.NET Web Application Template", then provide the Project a name as you wish and click on OK.
Choose MVC empty application option and click on OK
Step 2 - Add model class.
Right click on Model folder in the created MVC application and add class named City and right the following line of code,
City.cs
public class City
{
public int Id { get; set; }
public string Name { get; set; }
}
Step 3 Add user and admin controller
Right click on Controller folder in the created MVC application and add the controller class as,
Now after selecting controller template, click on add button then the following window appears,

Specify the controller name and click on add button, Now open the HomeController.cs file and write the following code into the Home controller class to bind and create the generic list from model class as in the following,
HomeController.cs
using System.Collections.Generic;
using System.Linq;
using System.Web.Mvc;
using AutoCompleteInMVCJson.Models;
namespace AutoCompleteInMVCJson.Controllers
{
public class HomeController : Controller
{
// GET: Home
[HttpGet]
public ActionResult Index()
{
return View();
}
[HttpPost]
public JsonResult Index(string Prefix)
{
//Note : you can bind same list from database
List<City> ObjList = new List<City>()
{
new City {Id=1,Name="Latur" },
new City {Id=2,Name="Mumbai" },
new City {Id=3,Name="Pune" },
new City {Id=4,Name="Delhi" },
new City {Id=5,Name="Dehradun" },
new City {Id=6,Name="Noida" },
new City {Id=7,Name="New Delhi" }
};
//Searching records from list using LINQ query
var Name = (from N in ObjList
where N.Name.StartsWith(Prefix)
select new { N.Name});
return Json(Name, JsonRequestBehavior.AllowGet);
}
}
}
In the above code, instead of going to database for records we are creating the generic list from model class and we will fetch records from above generic list.
Step 4
Reference jQuery UI css and js library reference as there are many ways to add the reference of jQuery library into the our project. The following are some methods:
Using NuGet package manager , you can install library and reference into the project
Use CDN library provided by Microsoft, jQuery, Google or any other which requires active internet connection.
Download jQuery files from jQuery official website and reference into the project.
In this example we will use jQuery CDN library.
Step 5
Create jQuery Ajax function to call controller JSON action method and invoke autocomplete function,
$(document).ready(function () {
$("#Name").autocomplete({
source: function(request,response) {
$.ajax({
url: "/Home/Index",
type: "POST",
dataType: "json",
data: { Prefix: request.term },
success: function (data) {
response($.map(data, function (item) {
return { label: item.Name, value: item.Name};
}))
}
})
},
messages: {
noResults: "", results: ""
}
});
})
To work above function don't forget to add the reference of the following jQuery CDN library as,
<link rel="stylesheet" href="//code.jquery.com/ui/1.11.4/themes/smoothness/jquery-ui.css">
<script src="//code.jquery.com/jquery-1.10.2.js"></script>
<script src="//code.jquery.com/ui/1.11.4/jquery-ui.js"></script>
Step 6
Add view named index and put above json function into the view. After adding code necessary files and logic the Index.cshtml will look like the following,
Index.cshtml
@model AutoCompleteInMVCJson.Models.City
@{
ViewBag.Title = "www.hostforlife.eu";
}
<link rel="stylesheet" href="//code.jquery.com/ui/1.11.4/themes/smoothness/jquery-ui.css">
<script src="//code.jquery.com/jquery-1.10.2.js"></script>
<script src="//code.jquery.com/ui/1.11.4/jquery-ui.js"></script>
<script type="text/javascript">
$(document).ready(function () {
$("#Name").autocomplete({
source: function (request, response) {
$.ajax({
url: "/Home/Index",
type: "POST",
dataType: "json",
data: { Prefix: request.term },
success: function (data) {
response($.map(data, function (item) {
return { label: item.Name, value: item.Name};
}))
}
})
},
messages: {
noResults: "", results: ""
}
});
})
</script>
@using (Html.BeginForm())
{
@Html.AntiForgeryToken()
<div class="form-horizontal">
<hr />
<div class="form-group">
<div class="col-md-12">
@Html.EditorFor(model => model.Name, new { htmlAttributes = new { @class = "form-control" } })
</div>
</div>
</div>
}
Now run the application and type any word then it will auto populate the records which exactly start with first word as in the following screenshot,
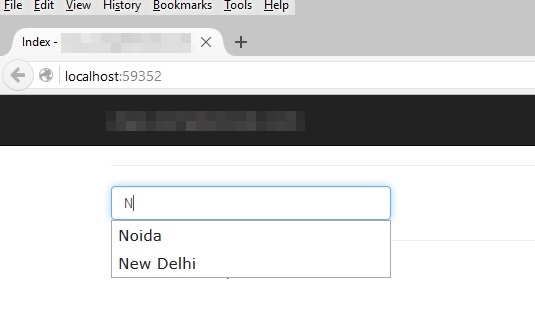
If you want to auto populate the records which contain any typed alphabet,then just change the LINQ query as contain. Now type any word it will search as follows,
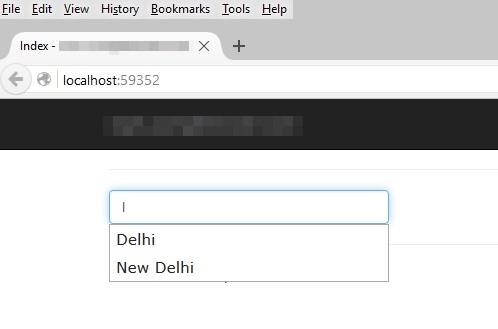
From all the above examples, I hope you learned how to create the auto complete textbox using jQuery UI in ASP.NET MVC.
Note
For the detailed code, please download the sample zip file.
Perform changes in Web.config file as per your server location.
You need to use the jQuery library.
For all the examples above, we learned how to use jQuery UI to create auto complete textbox in ASP.NET MVC. I hope this article is useful for all readers. If you have a suggestion then please contact me.