
August 7, 2023 10:56 by
Peter
Introduction: In this article, we will explore how to use AJAX to implement an edit functionality for student data in an ASP.NET MVC application. We'll demonstrate how to utilize jQuery AJAX to fetch the data from the server, populate an edit modal form, allow the user to make changes, and then update the database with the modified data. The provided code showcases a simple implementation that can serve as a foundation for more advanced data editing and updating tasks.
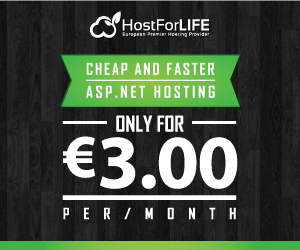
To follow along with this tutorial, you should have a basic understanding of ASP.NET MVC, C#, jQuery, and Entity Framework.
Overview of the Code
The code example consists of three main parts: the jQuery AJAX function in JavaScript, the ASP.NET MVC controller method, and the repository class responsible for database operations.
jQuery AJAX Function (Edit Function)
The editStudent(ID) function is responsible for making an AJAX request to the server to retrieve the student data for editing. It uses jQuery's $.ajax() method to send a GET request to the server, passing the student ID as a parameter.
function editStudent(ID) {
$.ajax({
url: '/Home/EditStudent/' + ID,
type: 'get',
success: function (response) {
$("#EditModal").html(response);
},
error: function (xhr, textStatus, error) {
// Handle error response
}
});
console.log("edit: " + ID);
}
ASP.NET MVC Controller Method
The HomeController class acts as the controller responsible for handling incoming requests. The EditStudent(int ID) action method is designed to receive the student ID sent by the AJAX function and fetch the student data from the repository.
public class HomeController : Controller
{
OperationRepositery repo = null;
public HomeController()
{
repo = new OperationRepositery();
}
public ActionResult EditStudent(int ID)
{
var result = repo.EditFunction(ID);
return View(result);
}
}
Repository Class (Edit Method)
The OperationRepositery class encapsulates the data access logic and implements the EditFunction(int ID) method to fetch the student data from the database using Entity Framework.
public class OperationRepositery
{
public StudentModel EditFunction(int ID)
{
using (var context = new StudentEntities())
{
var resultEdit = context.StudentTable.Where(x => x.ID == ID).Select(x => new StudentModel()
{
ID = x.ID,
Student = x.Student,
School = x.School,
Marks = x.Mark,
Rank = x.Rank,
Course = x.Stream,
Country = x.Country,
State = x.State,
City = x.City,
LanguageIds = x.Languages
}).FirstOrDefault();
return resultEdit;
}
}
}
In this article, we have learned how to use AJAX to implement the edit functionality for student data in an ASP.NET MVC application. The JavaScript AJAX function communicates with the server to fetch the data, and the ASP.NET MVC controller receives the request and retrieves the student data from the repository class. Developers can use this example as a starting point to implement more advanced features, such as validation, error handling, or additional data manipulation before updating the database. Remember to implement security measures and validate user input to protect the application from potential vulnerabilities. Happy coding!

August 4, 2023 07:40 by
Peter
In this tutorial, we will look at how to create a dynamic form with multiselect checkboxes in an ASP.NET MVC application. We'll concentrate on how to use jQuery AJAX to handle form submission, retrieve the selected checkboxes, and store the data to the database. The code provided offers a detailed example of how to develop a user-friendly student data management system that allows each student to select several languages.
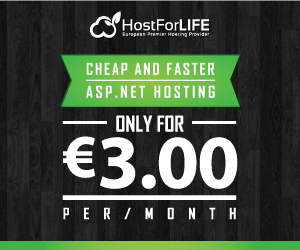
You should have a basic understanding of ASP.NET MVC, C#, jQuery, and Entity Framework before going.
How do you make a Multiselect Checkbox Form?
In the "Index.chtml" file, we have designed a form to collect student data, including a section to select multiple languages for each student using checkboxes.
<!-- Index.chtml -->
<!-- ... (existing code) ... -->
<div class="text-light text-center my-3">
<div class="d-flex justify-content-center">
<h5 class="text-warning">Languages:</h5>
<div id="languagesSection">
@for (int i = 0; i < Model.Languages.Count; i++)
{
<label>@Model.Languages[i].LanguageName</label>
@Html.CheckBoxFor(model => model.Languages[i].IsChecked)
@Html.HiddenFor(model => model.Languages[i].ID)
@Html.HiddenFor(model => model.Languages[i].LanguageName)
}
</div>
</div>
</div>
<!-- ... (existing code) ... -->
Implementing AJAX for Adding Data to Database
In the JavaScript section, we've implemented an AJAX function to handle the form submission and add student data to the database.
// AJAX for Adding Data to Database
$("#SumbitButton").click(function () {
var selected = []; // initialize array
$('div#languagesSection2 input[type=checkbox]').each(function () {
if ($(this).is(":checked")) {
selected.push($(this).attr("id"));
}
});
$("#Languages").val(selected);
var studentData = $("#studentInputForm").serialize();
if (!$('#studentInputForm').valid()) {
return false;
}
$.ajax({
url: "/Home/SaveStudent",
type: "POST",
data: studentData,
success: function (data) {
$('#studentInputForm').find(':input').val('');
$('input[type=checkbox]').prop('checked', false);
},
error: function (errormessage) {
console.log("error message");
}
});
});
Handling the AJAX Request in the Controller
In the "HomeController," we've added two action methods, one for saving student data and the other for handling the edit functionality.
public class HomeController : Controller
{
OperationRepositery repo = null;
public HomeController()
{
repo = new OperationRepositery();
}
public ActionResult Index()
{
StudentModel model = new StudentModel();
model.Languages = db.LanguagesTable.Select(lang => new LanguagesModel
{
ID = lang.ID,
LanguageName = lang.LanguagesName,
}).ToList();
return View(model);
}
// Action method for adding student data
public ActionResult SaveStudent(StudentModel model)
{
var result = repo.AddData(model);
return Json(new { result = true }, JsonRequestBehavior.AllowGet);
}
// Action method for handling the edit functionality
public ActionResult EditStudent(int ID)
{
var result = repo.EditFunction(ID);
var languageIdList = result.LanguageIds.Split(',').Select(x => Convert.ToInt32(x)).ToList();
result.Languages = db.LanguagesTable.Select(x => new LanguagesModel
{
ID = x.ID,
LanguageName = x.LanguagesName,
IsChecked = languageIdList.Contains(x.ID)
}).ToList();
return View(result);
}
}
Happy coding!

August 4, 2023 07:17 by
Peter
We will explore how to implement a dynamic form with multiselect checkboxes in an ASP.NET MVC application. We'll focus on using jQuery AJAX to handle the form submission, retrieve the selected checkboxes, and save the data to the database. The provided code includes a complete example of how to create a user-friendly student data management system with the ability to select multiple languages for each student.
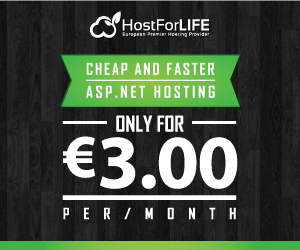
Before proceeding, you should have a basic understanding of ASP.NET MVC, C#, jQuery, and Entity Framework.
How to create the Multiselect Checkbox Form?
In the "Index.chtml" file, we have designed a form to collect student data, including a section to select multiple languages for each student using checkboxes.
<!-- Index.chtml -->
<!-- ... (existing code) ... -->
<div class="text-light text-center my-3">
<div class="d-flex justify-content-center">
<h5 class="text-warning">Languages:</h5>
<div id="languagesSection">
@for (int i = 0; i < Model.Languages.Count; i++)
{
<label>@Model.Languages[i].LanguageName</label>
@Html.CheckBoxFor(model => model.Languages[i].IsChecked)
@Html.HiddenFor(model => model.Languages[i].ID)
@Html.HiddenFor(model => model.Languages[i].LanguageName)
}
</div>
</div>
</div>
<!-- ... (existing code) ... -->
Implementing AJAX for Adding Data to Database
In the JavaScript section, we've implemented an AJAX function to handle the form submission and add student data to the database.
// AJAX for Adding Data to Database
$("#SumbitButton").click(function () {
var selected = []; // initialize array
$('div#languagesSection2 input[type=checkbox]').each(function () {
if ($(this).is(":checked")) {
selected.push($(this).attr("id"));
}
});
$("#Languages").val(selected);
var studentData = $("#studentInputForm").serialize();
if (!$('#studentInputForm').valid()) {
return false;
}
$.ajax({
url: "/Home/SaveStudent",
type: "POST",
data: studentData,
success: function (data) {
$('#studentInputForm').find(':input').val('');
$('input[type=checkbox]').prop('checked', false);
},
error: function (errormessage) {
console.log("error message");
}
});
});
Handling the AJAX Request in the Controller
In the "HomeController," we've added two action methods, one for saving student data and the other for handling the edit functionality.
public class HomeController : Controller
{
OperationRepositery repo = null;
public HomeController()
{
repo = new OperationRepositery();
}
public ActionResult Index()
{
StudentModel model = new StudentModel();
model.Languages = db.LanguagesTable.Select(lang => new LanguagesModel
{
ID = lang.ID,
LanguageName = lang.LanguagesName,
}).ToList();
return View(model);
}
// Action method for adding student data
public ActionResult SaveStudent(StudentModel model)
{
var result = repo.AddData(model);
return Json(new { result = true }, JsonRequestBehavior.AllowGet);
}
// Action method for handling the edit functionality
public ActionResult EditStudent(int ID)
{
var result = repo.EditFunction(ID);
var languageIdList = result.LanguageIds.Split(',').Select(x => Convert.ToInt32(x)).ToList();
result.Languages = db.LanguagesTable.Select(x => new LanguagesModel
{
ID = x.ID,
LanguageName = x.LanguagesName,
IsChecked = languageIdList.Contains(x.ID)
}).ToList();
return View(result);
}
}
Happy coding!