We can expose a Web API from ASP.NET MVC4. It is a new feature from Microsoft. Clients can get data from a Web API in any format such as JSON, XML, JSONP, HTML and etc.
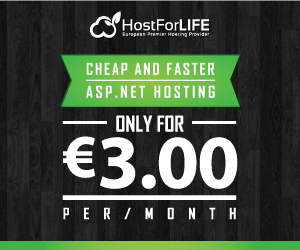
Now in this article, I am going to explain how to create a new Web API application and how to get data in various formats such as I mentioned above.
Purpose
HTTP is not just for serving up web pages. It is also a powerful platform for building APIs that expose services and data. HTTP is simple, flexible, and ubiquitous. Almost any platform that you can think of has an HTTP library, so HTTP services can reach a broad range of clients, including browsers, mobile devices, and traditional desktop applications.
The ASP.NET Web API is a framework for building web APIs on top of the .NET Framework. In this tutorial, you will use the ASP.NET Web API to create a web API that returns a list of products.
The following are the steps to create a new Web API application in Visual Studio 2011.
Step 1: We will create a new project and the project type will be MVC4. See the following image:
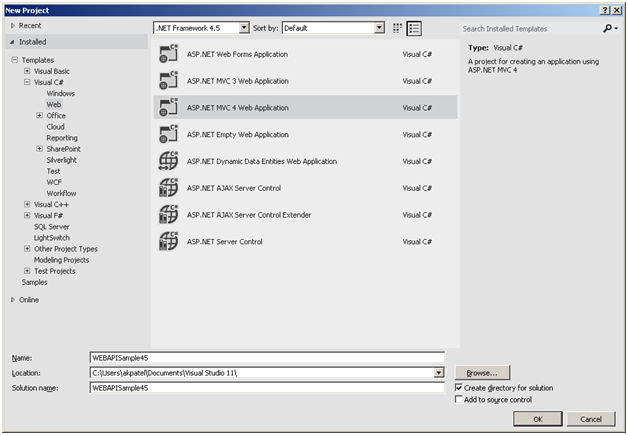
Step 2: Now we will select a Web API template from the Project template Dialog window.
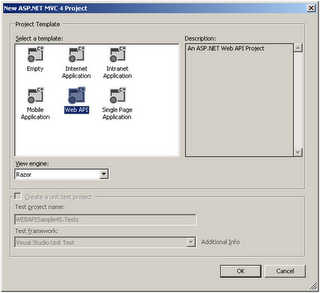
Step 3: Now we will create a Customer controller inside the Controller folder and will select an API controller with read/write actions.
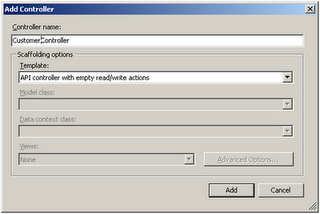
Step 4: Now we will add an Entity Data Model for our Database where we have a Customer Table. You can see it in the attached Source code.
Step 5: It's time to modify our CustomerController to fetch and save data. See the code below.
using System;
using System.Collections.Generic;
using System.Linq;
using System.Net.Http;
using System.Web.Http;
using WEBAPISample45.Models;
namespace WEBAPISample45.Controllers {
public class CustomerController: ApiController {
private CompanyDBEntities context = new CompanyDBEntities();
// GET /api/customer
public IEnumerable < CustomerModel > Get() {
IEnumerable < CustomerModel > list = null;
list = (from c in context.Customers select new CustomerModel { Id = c.Id, Name = c.Name, Salary = (long) c.Salary }
.AsEnumerable < CustomerModel > ();
return list;
}
// GET /api/customer/5
public CustomerModel Get(int id) {
return (from c in context.Customers where c.Id == id select new CustomerModel {
Id = c.Id,
Name = c.Name,
Salary = (long) c.Salary
}).FirstOrDefault < CustomerModel > ();
}
// POST /api/customer
public void Post(CustomerModel customer) {
context.AddToCustomers(new Customer {
Id = customer.Id, Name = customer.Name, Salary =
customer.Salary
});
context.SaveChanges(System.Data.Objects.SaveOptions.AcceptAllChangesAfterSave);
}
// PUT /api/customer/5
public void Put(int id, CustomerModel customer) {
var cust = context.Customers.First(c => c.Id == id);
cust.Name = customer.Name;
cust.Salary = customer.Salary;
context.SaveChanges();
}
// DELETE /api/customer/5
public void Delete(int id) {
var cust = context.Customers.First(c => c.Id == id);
context.Customers.DeleteObject(cust);
context.SaveChanges();
}
}
}
Step 7: Now we will run our application and see the output of the web API. Applications will run on the local server http://localhost:40683/ and you have to add the api/customer to the URL. So now your URL will be http://localhost:40683/api/customer.
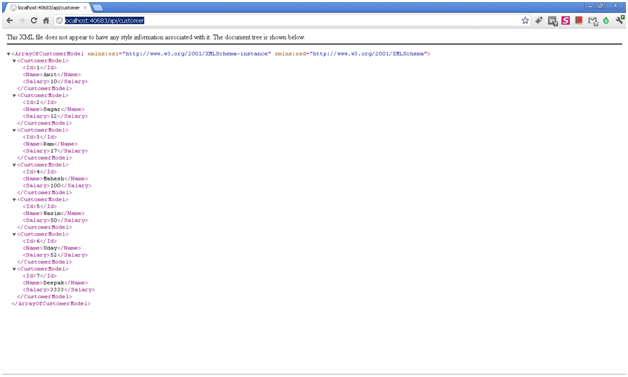
If you want to access a customer by id then you can use http://localhost:40683/api/customer/1 as the URL.
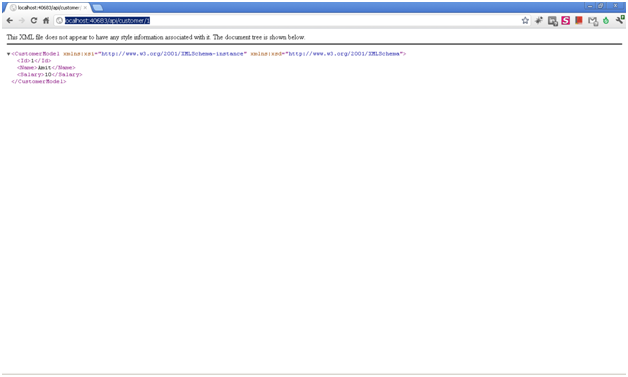
Now I am going to discuss a very important thing here. In the Web API, whatever format we use to send a request is the format of the returned response.
In the following JavaScript code, we are requesting data in JSON format. So the response will be returned in the JSON format. I will explain via Fiddler.
<script type="text/javascript">
$(document).ready(function () {
$.ajax({
type: "GET",
url: "api/customer/1",
dataType: "json",
success: function (data) {
alert(data);
}
});
});
</script>
XML
<script type="text/javascript">
$(document).ready(function () {
$.ajax({
type: "GET",
url: "api/customer/1",
dataType: "xml",
success: function (data) {
alert(data);
}
});
});
</script>
HTML
<script type="text/javascript">
$(document).ready(function () {
$.ajax({
type: "GET",
url: "api/customer/1",
dataType: "html",
success: function (data) {
alert(data);
}
});
});
</script>
If you see the above jQuery code we are only changing the DataType and we are not changing anything inside the Web API.
Now we will open Fiddler and see how we can get data in various formats.
Open Fiddler and go to the Composer tab. See the following screen:
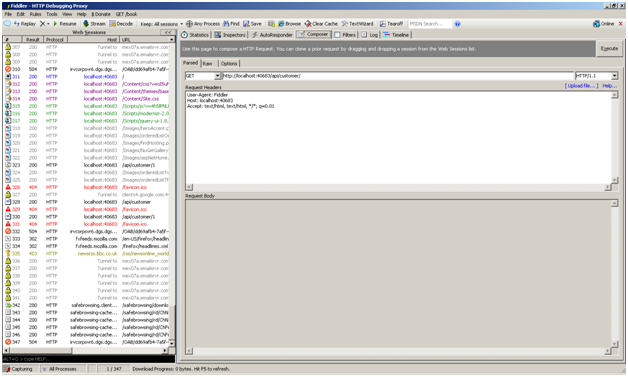
In the above image, you will see the "Accept: text/html, text/html, */*; q=0.01" when we execute the query it will return a response in HTML format.
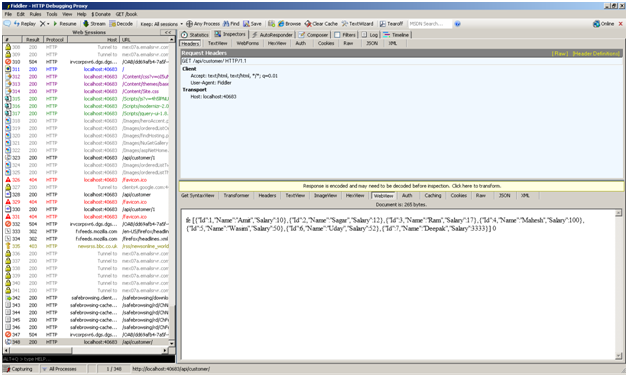
Now we will change the datatype to XML "Accept: application/xml, text/xml, */*; q=0.01" and will see the output in XML format.
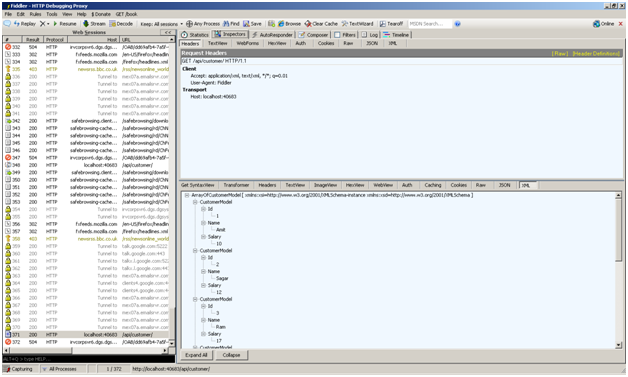
Now we will change the datatype to JSON "Accept: application/json, text/javascript, */*; q=0.01"
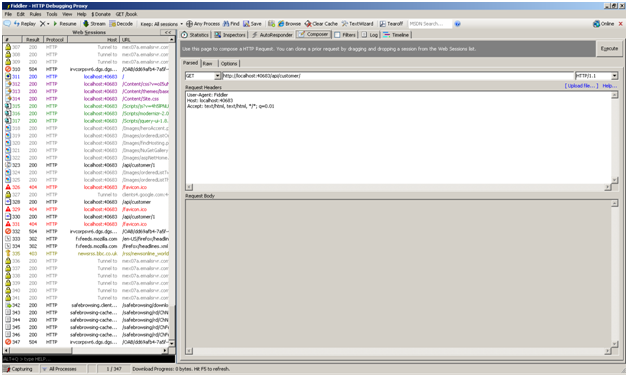
And the output is in JSON format:
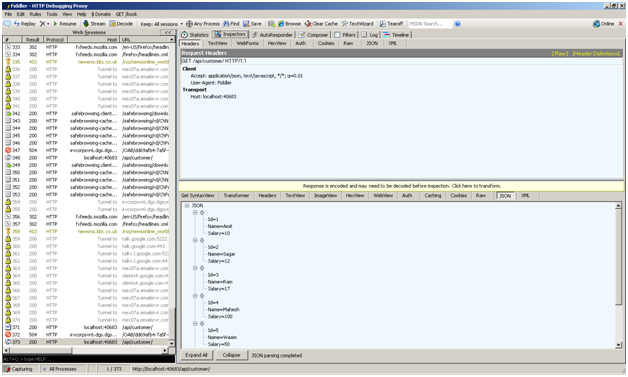