Harnessing the power of C# to enhance our data security through encryption and decryption is a versatile and necessary feature in the world of ASP.NET Core MVC. This security boost is made possible by the use of a variety of encryption methods, including but not limited to AES (Advanced Encryption Standard), RSA (Rivest-Shamir-Adleman), DES (Data Encryption Standard), and others. These cryptographic algorithms enable developers to protect sensitive information while keeping it secure and tamper-resistant.
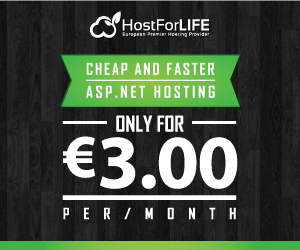
Let's look at how the AES algorithm, recognized for its strong encryption capabilities, may be smoothly integrated into our ASP.NET Core MVC application to encrypt and decrypt data. This example provides a baseline understanding of encryption processes within the ASP.NET Core MVC framework, allowing developers to begin exploring and implementing sophisticated security features in their web applications.
We'll have a better understanding of how to use the AES algorithm in our ASP.NET Core MVC projects by the end of this demonstration, enhancing our capacity to secure and protect key data assets. This knowledge enables us to make informed judgments about which encryption approaches best suit our application's particular security requirements, assuring the highest level of protection for our users' sensitive data.
Step 1: Create an Encryption/Decryption Helper Class.
To carry out encryption and decryption operations within our program, it is generally advised that we construct a specialized helper class. Here's an example of a well-structured class that can be utilized to improve the security of our application.
using System.Security.Cryptography;
namespace ZR.CodeExample.SecureMVC.Helpers
{
public static class EncryptionHelper
{
private static readonly string EncryptionKey = GenerateRandomKey(256);
public static string Encrypt(string plainText)
{
using (Aes aesAlg = Aes.Create())
{
aesAlg.Key = Convert.FromBase64String(EncryptionKey);
aesAlg.IV = GenerateRandomIV(); // Generate a random IV for each encryption
aesAlg.Padding = PaddingMode.PKCS7; // Set the padding mode to PKCS7
ICryptoTransform encryptor = aesAlg.CreateEncryptor(aesAlg.Key, aesAlg.IV);
using (MemoryStream msEncrypt = new MemoryStream())
{
using (CryptoStream csEncrypt = new CryptoStream(msEncrypt, encryptor, CryptoStreamMode.Write))
{
using (StreamWriter swEncrypt = new StreamWriter(csEncrypt))
{
swEncrypt.Write(plainText);
}
}
return Convert.ToBase64String(aesAlg.IV.Concat(msEncrypt.ToArray()).ToArray());
}
}
}
public static string Decrypt(string cipherText)
{
byte[] cipherBytes = Convert.FromBase64String(cipherText);
using (Aes aesAlg = Aes.Create())
{
aesAlg.Key = Convert.FromBase64String(EncryptionKey);
aesAlg.IV = cipherBytes.Take(16).ToArray();
aesAlg.Padding = PaddingMode.PKCS7; // Set the padding mode to PKCS7
ICryptoTransform decryptor = aesAlg.CreateDecryptor(aesAlg.Key, aesAlg.IV);
using (MemoryStream msDecrypt = new MemoryStream(cipherBytes, 16, cipherBytes.Length - 16))
{
using (CryptoStream csDecrypt = new CryptoStream(msDecrypt, decryptor, CryptoStreamMode.Read))
{
using (StreamReader srDecrypt = new StreamReader(csDecrypt))
{
return srDecrypt.ReadToEnd();
}
}
}
}
}
private static byte[] GenerateRandomIV()
{
using (Aes aesAlg = Aes.Create())
{
aesAlg.GenerateIV();
return aesAlg.IV;
}
}
private static string GenerateRandomKey(int keySizeInBits)
{
// Convert the key size to bytes
int keySizeInBytes = keySizeInBits / 8;
// Create a byte array to hold the random key
byte[] keyBytes = new byte[keySizeInBytes];
// Use a cryptographic random number generator to fill the byte array
using (var rng = new RNGCryptoServiceProvider())
{
rng.GetBytes(keyBytes);
}
// Convert the byte array to a base64-encoded string for storage
return Convert.ToBase64String(keyBytes);
}
}
}
This helper class contains the encryption and decryption functionality, as the name implies, making it easy to secure sensitive data in our ASP.NET Core MVC application. As part of best practices, we produce the encryption key dynamically. GenerateRandomKey(256)
We improve the security of our application by isolating the encryption and decryption code in a dedicated helper class. This method enables us to quickly handle sensitive data within our ASP.NET Core MVC application, adding an additional degree of security.
Step 2: Encryption and decryption are used in our controller or service
To use the encryption and decryption capabilities we've incorporated, we'll need to call the Encrypt and Decrypt methods within our controller or service class. Here's a detailed example on how to accomplish it.
using Microsoft.AspNetCore.Mvc;
using System.Diagnostics;
using ZR.CodeExample.SecureMVC.Helpers;
using ZR.CodeExample.SecureMVC.Models;
namespace ZR.CodeExample.SecureMVC.Controllers
{
public class HomeController : Controller
{
private readonly ILogger<HomeController> _logger;
public HomeController(ILogger<HomeController> logger)
{
_logger = logger;
}
public IActionResult Index()
{
// Define the data you want to secure
string plainText = "I am Peter from United Kingdom";
// Encrypt the data using the EncryptionHelper
string cipherText = EncryptionHelper.Encrypt(plainText);
// Decrypt the data to retrieve the original content
string decryptedText = EncryptionHelper.Decrypt(cipherText);
// Store the encrypted and decrypted data in ViewData for use in your view
ViewData["CipherText"] = cipherText;
ViewData["DecryptedText"] = decryptedText;
return View();
}
public IActionResult Privacy()
{
return View();
}
[ResponseCache(Duration = 0, Location = ResponseCacheLocation.None, NoStore = true)]
public IActionResult Error()
{
return View(new ErrorViewModel { RequestId = Activity.Current?.Id ?? HttpContext.TraceIdentifier });
}
}
}
Step 3: In the View, show both encrypted and decrypted data
One of the most impressive characteristics of Razor views is their ability to display both encrypted and decrypted data from our controller's ViewData dictionary. We can successfully provide sensitive information to our users in a secure manner by exploiting this feature. Let's look at an example of how this can be done within a Razor view.
@{
ViewData["Title"] = "Security ASP.net Core MVC (C#) Encryption and Decryption";
}
<div class="text-center">
<h1 class="display-4">@ViewData["Title"]</h1>
<p>By Peter, delve into the intricacies of security in ASP.NET Core MVC (C#) through our comprehensive article, focusing on the vital aspects of encryption and decryption techniques. Learn how to safeguard your web applications effectively.</p>
</div>
<div>
<h2>Encrypted Text:</h2>
<p>@ViewData["CipherText"]</p>
</div>
<div>
<h2>Decrypted Text:</h2>
<p>@ViewData["DecryptedText"]</p>
</div>
<div>
<h3>Who is Peter</h3>
<p>I am Peter, a seasoned Technical Lead Developer </p>
</div>
We'll suppose in this code snippet that our controller action is coupled with a view, such as Index.cshtml. We may display the encrypted and decrypted content on the web page by using this Razor view. Furthermore, we have the ability to modify the HTML structure and styling to match the design and requirements of our application.