
May 24, 2021 07:35 by
Peter
This article introduces how to display bootstrap alerts dynamically from an ASP.NET Core 3.1 MVC Application. Bootstrap provides us with an easy way to create predefined alerts. Bootstrap alerts are available for any length of text, as well as an optional close button. An example of a bootstrap alert is given below.
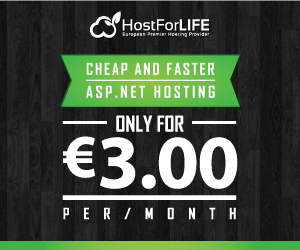
<div class="alert alert-success alert-dismissible">
<a href="#" class="close" data-dismiss="alert" aria-label="close">×</a>
<strong>Success!</strong> This alert box could indicate a successful or positive action.
</div>
Let's follow the below steps to show the alerts dynamically from a simple ASP.NET Core 3.1 MVC Application.
Step 1
Create an ASP.NET Core 3.1 MVC Web App.
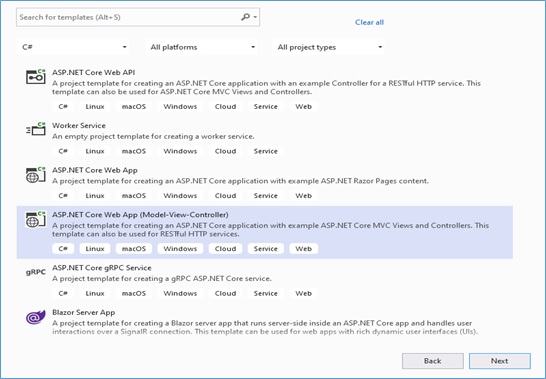
Step 2
Add a new folder and add an Enum into that folder and give a suitable name like this.
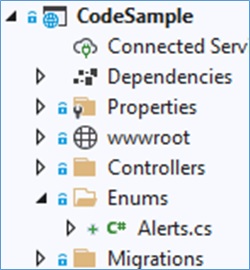
Step 3
Now create a common service like this.
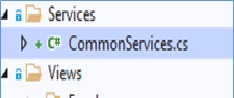
The code snippet for the CommonServices.cs class is given below. A new static method ‘ShowAlert’ is added here which takes two parameters and returns html as string.
public class CommonServices {
public static string ShowAlert(Alerts obj, string message) {
string alertDiv = null;
switch (obj) {
case Alerts.Success:
alertDiv = "<div class='alert alert-success alert-dismissable' id='alert'><button type='button' class='close' data-dismiss='alert'>×</button><strong> Success!</ strong > " + message + "</a>.</div>";
break;
case Alerts.Danger:
alertDiv = "<div class='alert alert-danger alert-dismissible' id='alert'><button type='button' class='close' data-dismiss='alert'>×</button><strong> Error!</ strong > " + message + "</a>.</div>";
break;
case Alerts.Info:
alertDiv = "<div class='alert alert-info alert-dismissable' id='alert'><button type='button' class='close' data-dismiss='alert'>×</button><strong> Info!</ strong > " + message + "</a>.</div>";
break;
case Alerts.Warning:
alertDiv = "<div class='alert alert-warning alert-dismissable' id='alert'><button type='button' class='close' data-dismiss='alert'>×</button><strong> Warning!</strong> " + message + "</a>.</div>";
break;
}
return alertDiv;
}
}
Now let’s call this method from a controller which is saving records in the database. The following is the code snippet for the controller.
// POST: EmployeeController/Create
[HttpPost]
[ValidateAntiForgeryToken]
public ActionResult Create([FromForm] EmployeeViewModel collection) {
try {
int result = 0;
if (ModelState.IsValid) {
Employee objEmp = new Employee();
objEmp.EmpName = collection.EmpName;
objEmp.Address = collection.Address;
objEmp.Email = collection.Email;
objEmp.Phone = collection.Phone;
objEmp.BankAccountNo = collection.BankAccountNo;
objEmp.CreatedOn = DateTime.Now;
objEmp.CreatedBy = "SYSTEM";
objEmp.ModifiedOn = null;
objEmp.ModifiedBy = null;
result = _empRepo.Add(objEmp).Result;
//return RedirectToAction("Index");
if (result > 0) {
ViewBag.Alert = CommonServices.ShowAlert(Alerts.Success, "Employee added");
} else ViewBag.Alert = CommonServices.ShowAlert(Alerts.Danger, "Unknown error");
}
return PartialView("_Create");
} catch {
return View();
}
}
Code Explanation
In post type method we pass EmployeeViewModel class as a parameter as a model name that gets all data from the user.
_empRepo.Add() is a custom method used to save the Employee records. ( Repository Pattern is used to save a record here. You can download complete source code from the GitHub link given below ).
Step 4
Now add the below line in the view like this. It helps to render the HTML from the ViewBag string returned from the static method.
@Html.Raw(@ViewBag.Alert)
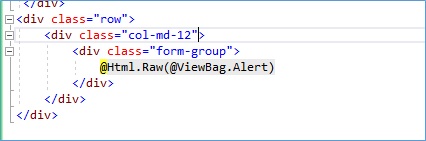
The following is the complete code snippet for the view.
@model CodeSample.Models.EmployeeViewModel
@{
ViewData["Title"] = "Add Employee";
Layout = "~/Views/Shared/_Layout.cshtml";
}
<h4>Add Employee</h4>
<hr />
<div class="row">
<div class="col-md-4">
<form asp-action="Create" asp-controller="Employee" method="post">
<div asp-validation-summary="ModelOnly" class="text-danger"></div>
<div class="form-group">
<label asp-for="EmpName" class="control-label">Enter employee name</label>
<input asp-for="EmpName" class="form-control" />
<span asp-validation-for="EmpName" class="text-danger"></span>
</div>
<div class="form-group">
<label asp-for="Email" class="control-label">Enter email</label>
<input asp-for="Email" class="form-control" />
<span asp-validation-for="Email" class="text-danger"></span>
</div>
<div class="form-group">
<label asp-for="Phone" class="control-label">Enter Phone No.</label>
<input asp-for="Phone" class="form-control" />
<span asp-validation-for="Phone" class="text-danger"></span>
</div>
<div class="form-group">
<label asp-for="Address" class="control-label">Enter Address</label>
<textarea asp-for="Address" class="form-control"></textarea>
<span asp-validation-for="Address" class="text-danger"></span>
</div>
<div class="form-group">
<label asp-for="BankAccountNo" class="control-label">Enter Bank Account No.</label>
<input asp-for="BankAccountNo" class="form-control" />
<span asp-validation-for="BankAccountNo" class="text-danger"></span>
</div>
<div class="form-group">
<input type="submit" id="SaveEmp" value="Create" class="btn btn-primary" />
</div>
</form>
</div>
</div>
<div class="row">
<div class="col-md-12">
<div class="form-group">
@Html.Raw(@ViewBag.Alert)
</div>
</div>
</div>
<div><a asp-action="Index">Back to List</a></div>
@section Scripts {
@{await Html.RenderPartialAsync("_ValidationScriptsPartial");}
}
Now if something goes wrong and the application is unable to insert the record in the database, it will show an error alert like this.
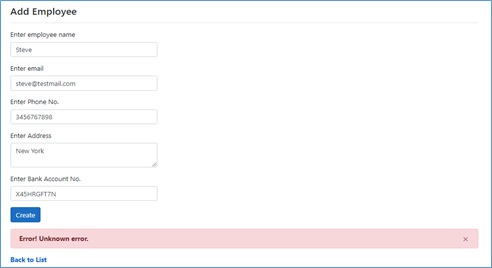
Else it will show a success alert like this.
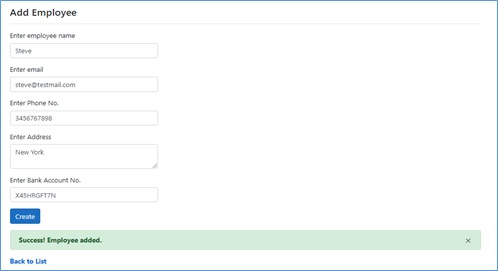
Here we saw how to display a bootstrap alert dynamically from ASP.NET Core 3.1 MVC Application. Hope you like this article and get some information from it.