
July 25, 2024 09:28 by
Peter
We can regulate the session state behavior in ASP.NET MVC with the aid of the SessionState Attribute. Using this property, we may set the session state to read-only, required, or disabled for the controller. We can only use this attribute at the controller level because it is a class level attribute. A controller's action methods may behave differently from the controller session state behavior in some cases. The following solution is quite helpful in this situation. Thus, in ASP.NET MVC, we may implement a session state behavior for each action.
Problem Synopsis
The SessionState property allows us to regulate the behavior of the session state, however it is only applicable at the controller level. This indicates that the session state behavior is the same for all of the controller's action methods. What is the course of action to take now that certain controller action methods do not require a session while others do?
Resolution
In this case, all the action methods that share the same session state behavior can be moved to a distinct controller class and made. This is a poor course of action. Alternatively, we may override the session state behavior for that particular action method by creating a custom action property.
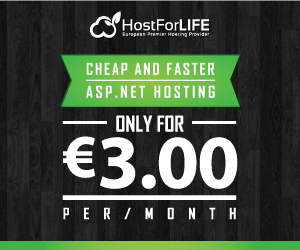
To create a custom action attribute that overrides the session state's behavior, follow these steps.
First, make a custom attribute.
[AttributeUsage(AttributeTargets.Method, AllowMultiple = false, Inherited = true)]
public sealed class ActionSessionStateAttribute : Attribute
{
public SessionStateBehavior Behavior { get; private set; }
public ActionSessionStateAttribute(SessionStateBehavior behavior)
{
this.Behavior = behavior;
}
}
Step 2: Create custom controller factory
public class CustomControllerFactory : DefaultControllerFactory
{
protected override SessionStateBehavior GetControllerSessionBehavior(RequestContext requestContext, Type controllerType)
{
if (controllerType == null)
{
return SessionStateBehavior.Default;
}
var actionName = requestContext.RouteData.Values["action"].ToString();
MethodInfo actionMethodInfo;
actionMethodInfo = controllerType.GetMethod(actionName, BindingFlags.IgnoreCase | BindingFlags.Public | BindingFlags.Instance);
if (actionMethodInfo != null)
{
var actionSessionStateAttr = actionMethodInfo.GetCustomAttributes(typeof(ActionSessionStateAttribute), false)
.OfType<ActionSessionStateAttribute>()
.FirstOrDefault();
if (actionSessionStateAttr != null)
{
return actionSessionStateAttr.Behavior;
}
}
return base.GetControllerSessionBehavior(requestContext, controllerType);
}
}
Step 3: Register custom controller factory in Global.asax
protected void Application_Start()
{
AreaRegistration.RegisterAllAreas();
RegisterGlobalFilters(GlobalFilters.Filters);
RegisterRoutes(RouteTable.Routes);
ControllerBuilder.Current.SetControllerFactory(typeof(CustomControllerFactory));
}
Step 4: Attribute usages
[SessionState(System.Web.SessionState.SessionStateBehavior.Disabled)]
public class HomeController : Controller
{
public ActionResult Index()
{
ViewBag.Message = "Welcome to ASP.NET MVC!";
TempData["test"] = "session less controller test";
return View();
}
[ActionSessionState(System.Web.SessionState.SessionStateBehavior.Required)]
public ActionResult About()
{
Session["test"] = "session less controller test";
return View();
}
}