An overview of MVC, or Model View Controller
A framework called Model View Controller (MVC) is used to create web applications based on predetermined designs.
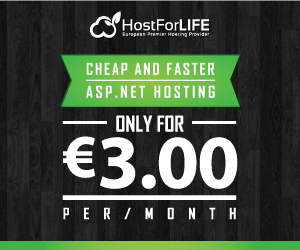
- M is an acronym for model.
- V is an acronym for view.
- Controller is represented by the letter C.
The portion of the program that manages the logic for application data is represented by ModelModel. The Model object's primary functions are data retrieval and database storage.
Views
The portion of a program that manages data display is called a view. Model data are used to construct views.
Controller
The portion of the program that manages user interaction is called the Controller. It serves as a link between the View and the Model. Usually, the Controller provides input data to the model after reading data from the View and controlling user input.
What has ASP.NET MVC 6 added?
Cloud-optimized Framework vs. Entire Framework
Because System.Web.Dll is so expensive and often uses 30k of memory for each request and answer, Microsoft eliminated its reliance from MVC 6. As a result, MVC 6 now only uses 2k of memory for requests and responses, which is a relatively minimal amount of memory. One benefit of utilizing the cloud-optimized framework is that your website will come with a copy of the mono CLR. We don't need to update the.NET version on the whole system only for one website. A separate CLR version operating side by side with another website.
ASP.NET 5's MVC 6 component was created with cloud-optimized apps in mind. When our MVC application is pushed to the cloud, the runtime chooses the appropriate version of the library automatically.
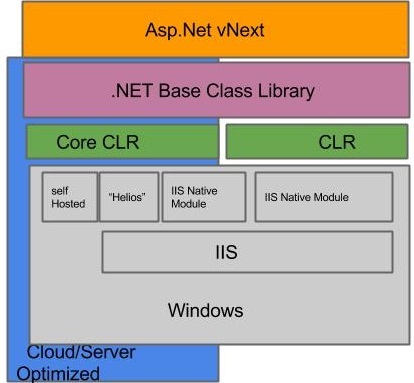
It is also intended to optimize the Core CLR with a high degree of resource efficiency.
Microsoft created numerous Web API, WebPage, MVC, and SignalLr components that we refer to as MVC 6.
The Roslyn Compiler is used to solve the majority of the difficulties. The Roslyn Compiler is used with ASP.NET vNext. We can avoid compiling the program by using the Roslyn Compiler, which builds the application code automatically. Without pausing or restarting the project, you will update a code file and be able to view the changes by refreshing the browser.
Use hosts other than Windows
On the other hand, MVC 6 has an improved functionality that is housed on an IIS server and a self-user pipeline. Where we use MVC5, we can host it on an IIS server and we can also run it on top of an ASP. Net Pipeline.
Configuration system based on the environment
An environment for cloud application deployment is made available via the configuration system. Our program functions similarly to one that provides configurations. Retrieving the value from other configuration sources, such as XML files, is helpful.
A brand-new environment-based configuration system is part of MVC6. It is dependent only on the Web, as opposed to something else. Configuration file from the prior iteration.
Dependency injection
Using the IServiceProvider interface we can easily add our own dependency injection container. We can replace the default implementation with our own container.
Supports OWIN
We have complete control over the composable pipeline in MVC 6 applications. MVC 6 supports the OWIN abstraction.
Important components of an MVC6 application
The is a new file type in MVC6 as in the following.
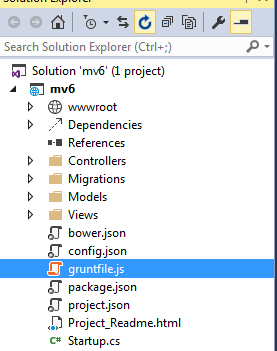
The preceding files are new in MVC 6. Let's see what each of these files contains.
Config.Json
This file contains the application configuration in various places. We can define our application configuration, not just this file. There is no need to be concerned about how to connect to various sources to get the confutation value.
In the following code, we add a connection string in the Config.json file.
{
"Data": {
"DefaultConnection": {
"ConnectionString": "Server=server_name;Database=database_name;Trusted_Connection=True;MultipleActiveResultSets=true"
}
}
}
Project.json
This file contains the build information as well as project dependencies. It can contain the commands used by the application.
{
"webroot": "wwwroot",
"version": "1.0.0-*",
"dependencies": {
"Microsoft.AspNet.Mvc": "6.0.0-beta1",
"Microsoft.AspNet.Server.IIS": "1.0.0-alpha4"
},
"commands": {
/* Change the port number when you are self hosting this application */
"web": "Microsoft.AspNet.Hosting --server Microsoft.AspNet.Server.WebListener --server.urls http://localhost:5000"
},
"frameworks": {
"aspnet50": {},
"aspnetcore50": {}
}
}
Startup.cs
The Application Builder is used as a parameter when the configure method is used when the Startup class is used by the host.
Global.json
Define the location for the project reference so that the projects can reference each other.
Defining the Request Pipeline
If we look at the Startup.cs it contains a startup method.
public Startup(IHostingEnvironment env)
{
// Setup configuration sources.
var Configuration = new Configuration();
Configuration.AddJsonFile("config.json");
Configuration.AddEnvironmentVariables();
}
Create a Controller
If we look at the controller it is similar to the controller in a MVC 5 application.
public class HomeController : Controller
{
public IActionResult Index()
{
return View();
}
}
The namespace for MVC6 is Microsoft.ASPNET.MVC, unlike the System.Web.MVC in the previous version. That is a big difference. In MVC 6 the application does not need to be derived from the controller class. In our controller, there are many default functionalities. Most of the time we might need to derive from the controller class, but if we do not need access to all of the functionally provided by the controller class, we can define our controller class.
public class HomeController : Controller
{
public ActionResult Index()
{
return View();
}
public string atul()
{
return "hello i am mvc6";
}
}
If you execute the preceding method we may see the following page.
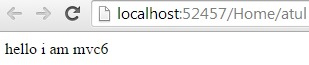
Summary
In this article, we have learned about the new features of MVC 6. We have now become familiar with MVC 6.