Creating, reading, updating, and deleting (CRUD) operations are essential functionalities for any web application. In this article, we'll explore how to implement these operations in an ASP.NET MVC application using Razor views and C#.
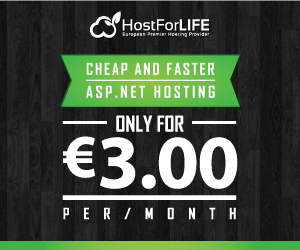
Controller Actions
Democurd Action
The democurd action is responsible for displaying a list of democurd records.
public ActionResult democurd()
{
DemoModel model = new DemoModel();
model.Id = Convert.ToInt32(Session["Id"]);
var list = demovider.Getdemocurd(model);
return View(list);
}
- Initialization: A DemoModel object is created and its Id property is set from a session variable.
- Data Fetching: The Getdemocurd method of demovider is called to fetch a list of democurd records.
- Return View: The list of records is passed to the view for display.
democurdAddEdi Action (GET)
The democurdAddEdi action displays a form for adding or editing a democurd record.
public ActionResult democurdAddEdi()
{
democurdinput model = new democurdinput();
return View(model);
}
Model Initialization: A new democurdinput model is initialized.
Return View: The model is passed to the view for rendering the form.
democurdAddEdi Action (POST)
This action handles the form submission for adding or editing a democurd record.
[HttpPost]
[AllowAnonymous]
[ValidateAntiForgeryToken]
public async Task<ActionResult> democurdAddEdi(democurdinput obj)
{
try
{
if (ModelState.IsValid)
{
// Your logic here
return RedirectToAction("StationMaster");
}
else if (obj.StationId != 0)
{
var Li = democurdAddvider.democurdAdd(obj);
if (Li.Status == "0")
{
return RedirectToAction("democurd");
}
else
{
return View(obj);
}
}
}
catch (Exception ex)
{
// Handle exception
}
return View(obj);
}
- Form Validation: Checks if the model state is valid.
- Condition Handling: Depending on the StationId, it either redirects or returns the view with validation errors.
- Exception Handling: Catches and handles any exceptions that occur during the process.
Editdemocurd Action
The Editdemocurd action fetches a democurd record for editing.
public ActionResult Editdemocurd(int? id)
{
democurdinput model = new democurdinput();
try
{
model.dmId = Convert.ToInt32(id);
var list = democurdvider.Getdemocurd(model);
var ass = list.FirstOrDefault();
return PartialView("StationMasterAddEdi", ass);
}
catch (Exception ex)
{
// Handle exception
}
return View("democurdAddEdi", null);
}
Model Initialization: A new democurdinput model is initialized with the id parameter.
Data Fetching: Retrieves the record to be edited using the Getdemocurd method.
Return Partial View: The record is passed to a partial view for editing.
<div class="row">
<div class="col-md-12">
<div class="card">
<div class="card-body">
<div class="card-title">
<i class="icon-layers"></i> Manage democurd
<span class="pull-right">
<a href="@Url.Action("methodname", "controllername")" class="btn btn-primary mt-ladda-btn ladda-button btn-circle" data-style="expand-right">
<span class="ladda-label">
<i class="fa fa-plus"></i> Add
</span>
</a>
</span>
</div>
<div class="preview-list">
<table class="table table-striped table-bordered dt-responsive nowrap" id="dataTableModules1">
<thead>
<tr>
<th>Name</th>
<th>Number</th>
<th>val</th>
<th>valtude</th>
<th>status</th>
<th>StatusDesc</th>
<th>wstg</th>
<th class="text-center">Action</th>
</tr>
</thead>
<tbody>
@foreach (var i in Model)
{
<tr>
<td>@i.Name</td>
<td>@i.Number</td>
<td>@i.val</td>
<td>@i.valtude</td>
<td>@i.status</td>
<td>@i.StatusDesc</td>
<td>@i.wstg</td>
<td class="text-center">
<a href="@Url.Action("Editdemocurd", "Master", new { id = @i.Id })" class="btn btn-success btn-fw" data-style="expand-right">
<span class="ladda-label">
<i class="fa fa-pencil-square-o"></i>
</span>
</a>
</td>
</tr>
}
</tbody>
</table>
</div>
</div>
</div>
</div>
</div>
Table Structure: The table includes columns for various attributes of the democurd records and an action column for editing.
Add Button: A button to add a new record, redirecting to the StationMasterAddEdi action.
JavaScript for DataTable and Alerts
JavaScript code to initialize DataTable and display success or failure alerts.
@section scripts {
@{
if (TempData["AlertError"] != null)
{
<script>
swal("Failure", "@TempData["AlertError"].ToString()", "error");
</script>
}
if (TempData["AlertSuccess"] != null)
{
<script>
swal("Success", "@TempData["AlertSuccess"].ToString()", "success");
</script>
}
}
}
<script>
$(document).ready(function () {
window.showLoading({ name: 'jump-pulse', allowHide: true });
$('#dataTableModules1').dataTable({
autoWidth: false,
responsive: true,
"bLengthChange": true,
"bFilter": true,
"language": {
"searchPlaceholder": "Search"
},
"aaSorting": [],
"sPaginationType": "full_numbers",
dom: 'Bfrtip',
buttons: [
{
extend: 'copyHtml5',
text: '<i class="fa fa-files-o"></i> Copy',
titleAttr: 'Copy'
},
{
extend: 'excelHtml5',
text: '<i class="fa fa-file-excel-o"></i> Excel',
titleAttr: 'Excel'
},
{
extend: 'csvHtml5',
text: '<i class="fa fa-file-text-o"></i> CSV',
titleAttr: 'CSV'
},
{
extend: 'pdfHtml5',
text: '<i class="fa fa-file-pdf-o"></i> PDF',
titleAttr: 'PDF'
}
]
});
setTimeout(function () {
window.hideLoading();
}, 1000);
});
</script>
- Alerts: Displays success or failure alerts based on TempData values.
- DataTable Initialization: Initializes the DataTable with specific options and buttons for exporting data.
Form for Adding or Editing democurd Records
A form for adding or editing democurd records with validation and anti-forgery protection.
@using (Html.BeginForm("democurdAddEdi", "democontroller", FormMethod.Post, new { id = "__AjaxAntiForgeryForm", role = "form", LoadingElementId = "please-wait" }))
{
@Html.AntiForgeryToken()
<div class="form-body">
<div class="row">
<div class="col-md-3">
<div class="form-group form-md-line-input form-md-floating-label">
<label for="form_control_1">Station Name<span class="text-danger">*</span></label>
<div class="input-icon right">
@Html.TextBoxFor(m => m.Name, new { placeholder = "Enforcement Name", @class = "form-control", onkeypress = "return onlyAlphabets(this, event);", maxlength = "100", @required = true })
@Html.HiddenFor(m => m.StationId)
<span class="help-block">@Html.ValidationMessageFor(m => m.Name, "", new { @class = "text-danger", style = "white-space:nowrap" })</span>
</div>
</div>
</div>
<div class="col-md-3">
<div class="form-group form-md-line-input form-md-floating-label">
<label for="form_control_1">Mobile Number<span class="text-danger">*</span></label>
<div class="input-icon right">
@Html.TextBoxFor(m => m.Number, new { placeholder = "Number", @class = "form-control", onkeypress = "return onlyNumber(this, event);", maxlength = "10", minlength = "10", @required = true })
<span class="help-block">@Html.ValidationMessageFor(m => m.Number, "", new { @class = "text-danger", style = "white-space:nowrap" })</span>
</div>
</div>
</div>
<div class="col-md-3">
<div class="form-group form-md-line-input form-md-floating-label">
<label for="form_control_1">val<span class="text-danger">*</span></label>
<div class="input-icon right">
@Html.TextBoxFor(m => m.val, new { placeholder = "val", @class = "form-control", onkeypress = "return onlyNumberwithDot(this, event);", maxlength = "100", @required = true })
<span class="help-block">@Html.ValidationMessageFor(m => m.val, "", new { @class = "text-danger", style = "white-space:nowrap" })</span>
</div>
</div>
</div>
<div class="col-md-3">
<div class="form-group form-md-line-input form-md-floating-label">
<label for="form_control_1">valtude<span class="text-danger">*</span></label>
<div class="input-icon right">
@Html.TextBoxFor(m => m.valtude, new { placeholder = "valtude", @class = "form-control", onkeypress = "return onlyNumberwithDot(this, event);", maxlength = "100", @required = true })
<span class="help-block">@Html.ValidationMessageFor(m => m.valtude, "", new { @class = "text-danger", style = "white-space:nowrap" })</span>
</div>
</div>
</div>
</div>
<div class="row">
<div class="col-md-3">
<div class="form-group form-md-line-input form-md-floating-label">
<label for="form_control_1">status</label>
<div class="input-icon right">
@Html.TextBoxFor(m => m.status, new { placeholder = "status", @class = "form-control", @required = true })
<span class="help-block">@Html.ValidationMessageFor(m => m.status, "", new { @class = "text-danger", style = "white-space:nowrap" })</span>
</div>
</div>
</div>
<div class="form-actions noborder pull-right">
<a href="@Url.Action("methodname", "controllername")" class="btn btn-danger btn-flat"><i class="fa fa-ban fa-fw"></i>Cancel</a>
<button id="btnSubmit" type="submit" class="btn btn-info btn-flat"><i class="fa fa-save fa-fw"></i>Submit</button>
</div>
</div>
</div>
}
Conclusion
In this article, we've covered the implementation of CRUD operations in an ASP.NET MVC application using Razor views and C#. We explored the various controller actions responsible for listing, adding, and editing records. Additionally, we discussed the Razor views for displaying the records in a table and providing forms for user input.