
September 4, 2024 08:36 by
Peter
Attribute routing is a potent feature in ASP.NET Core that lets you explicitly define routing information on controller activities. Compared to traditional routing, this offers more flexibility and control over how HTTP requests are routed to your API endpoints. This is a summary of attribute routing's functions and how to use it in your.NET Core API.
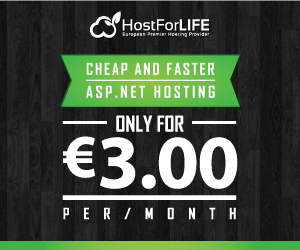
Attribute Routing: What is It?
With attribute routing, you may use attributes to construct routes directly on your action methods or controllers. This method can help make routing easier to comprehend and control because it is more explicit.
How to use Attribute Routing?
1. Basic Attribute Routing
You can apply routing attributes directly to action methods and controllers. For example.
[ApiController]
[Route("api/[controller]")]
public class ProductsController : ControllerBase
{
// GET api/products
[HttpGet]
public IActionResult GetAllProducts()
{
// Implementation
}
// GET api/products/5
[HttpGet("{id}")]
public IActionResult GetProductById(int id)
{
// Implementation
}
// POST api/products
[HttpPost]
public IActionResult CreateProduct([FromBody] Product product)
{
// Implementation
}
}
Controller Route: [Route("api/[controller]")] sets the base route for all actions in the controller. [controller] is a placeholder that gets replaced with the name of the controller (e.g., Products).
Action Routes: [HttpGet], [HttpGet("{id}")], [HttpPost] specify the HTTP methods and the routes for the actions.
2. Route Parameters
You can use route parameters in the attribute routing to capture values from the URL.
[HttpGet("search/{query}")]
public IActionResult SearchProducts(string query)
{
// Implementation
}
Route Parameter: {query} in the route template will capture the value from the URL and pass it to the action method.
3. Optional Parameters
To make route parameters optional, use the following syntax.
[HttpGet("items/{id?}")]
public IActionResult GetItemById(int? id)
{
// Implementation
}
Optional Parameter: {id?} makes the id parameter optional.
4. Route Constraints
You can add constraints to route parameters to enforce specific patterns.
[HttpGet("products/{id:int}")]
public IActionResult GetProductById(int id)
{
// Implementation
}
Route Constraint: {id:int} ensures that the id parameter must be an integer.
5. Route Prefixes and Constraints at Controller Level
You can also set route prefixes and constraints at the controller level.
[ApiController]
[Route("api/[controller]/[action]")]
public class OrdersController : ControllerBase
{
[HttpGet]
public IActionResult GetAllOrders()
{
// Implementation
}
[HttpGet("{id:int}")]
public IActionResult GetOrderById(int id)
{
// Implementation
}
}
Action Route: [action] is replaced with the action method name in the route URL.
6. Combining Routes
You can combine routes and use route templates to create complex routing scenarios.
[ApiController]
[Route("api/[controller]")]
public class CustomersController : ControllerBase
{
[HttpGet("{customerId}/orders/{orderId}")]
public IActionResult GetCustomerOrder(int customerId, int orderId)
{
// Implementation
}
}
Benefits of Attribute Routing
Clarity and Control: Route definitions are more explicit and closely related to their corresponding actions, making it easier to understand and manage routes.
Flexibility: Allows for more complex routing scenarios, including optional and default parameters, constraints, and combined routes.
Customization: Facilitates precise control over route templates and endpoints.
Combined Route
The route combines customerId and orderId parameters to define a more specific endpoint.