
August 26, 2024 06:55 by
Peter
Several forms to manage modal popups can result in repetitive code and more maintenance work. We'll look at how to centralize modal popup code in a.NET MVC application in this blog post. This will let you define the modal once and utilize it in other places in your project. We will cover how to create a modal's partial view, add it to various sites, and effectively handle modal triggers. You will have a better structured and manageable method for dealing with modal popups in your.NET MVC apps at the end of this article.
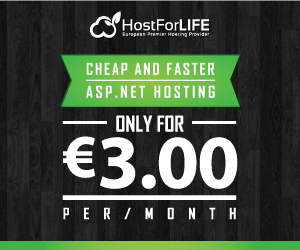
You can centralize the modal popup code and utilize it throughout your application to avoid repeating it in every form. Here's how to go about it.
Establish a Partial Model View
The modal code can be included in a partial view that you design. You can add this partial view to any page that requires a modal.
Steps
Create Partial View
Right-click on the Views/Shared folder (or any other folder) and select Add -> View.
Name the view _DeleteModal.cshtml.
In the view, add the modal code.
<div class="modal-dialog">
<div class="modal-content">
<div class="modal-header">
<h5 class="modal-title">
<asp:Label ID="ltrlDelHead" Text="Are You Sure, You want to Delete This?" runat="server"></asp:Label>
</h5>
<button type="button" class="bootbox-close-button close" data-dismiss="modal" aria-hidden="true">×</button>
</div>
<div class="modal-body">
<div class="bootbox-body">
<div class="bootbox-form"></div>
</div>
</div>
<div class="modal-footer">
<button type="button" class="btn btn-link" data-dismiss="modal">Cancel</button>
<asp:LinkButton ID="lnkRecordDelete" class="btn bg-danger" runat="server" OnClick="lnkRecordDelete_Click">Delete</asp:LinkButton>
</div>
</div>
</div>
Include Partial View in Your Pages
In any view where you want to use this modal, simply include the partial view.
@Html.Partial("_DeleteModal")
Alternative Using Layout or Master Page
If you need this modal on many pages, you can add the modal code directly to your layout or master page so it’s available throughout your application.
Steps
Open your Layout.cshtml (or master page if using WebForms).
Add the modal code before the closing </body> tag.
<div class="modal fade" id="deleteModal" tabindex="-1" role="dialog" aria-hidden="true">
@Html.Partial("_DeleteModal")
</div>
Then, use JavaScript or server-side logic to trigger this modal when needed.
Triggering the Modal
To trigger the modal from different parts of your application, you can use JavaScript or server-side code to open it when required.
$('#deleteModal').modal('show');
This approach centralizes the modal code, making your application more maintainable and easier to manage. Now, you only need to update the modal in one place if any changes are required.