
January 28, 2022 06:27 by
Peter
Routing is a pattern matching process that monitors the requests and determines what to do with each request. In other words we can say Routing is a mechanism for mapping requests within our MVC application.
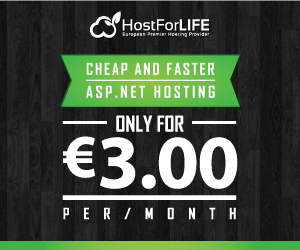
When a MVC application starts the first time, the Application_Start () event of global.asax is called. This event registers all routes in the route table using the RouteCollection.MapRoute method.
Example
routes.MapRoute(
"Default", // Route name
"{controller}/{action}/{id}", // URL with parameters
new { controller = "Home", action = "about", id = UrlParameter.Optional } // Parameter defaults
);
MVC 5 supports a new type of routing called “Attribute Routing”. As the name suggests, Attribute Routing enables us to define routing on top of the controller action method.
Enabling Attribute Routing
To enable Attribute Routing, we need to call the MapMvcAttributeRoutes method of the route collection class during configuration.
public class RouteConfig
{
public static void RegisterRoutes(RouteCollection routes)
{
routes.IgnoreRoute("{resource}.axd/{*pathInfo}");
routes.MapMvcAttributeRoutes();
}
}
We can also add a customized route within the same method. In this way we can combine Attribute Routing and convention-based routing.
public class RouteConfig
{
public static void RegisterRoutes(RouteCollection routes)
{
routes.IgnoreRoute("{resource}.axd/{*pathInfo}");
routes.MapMvcAttributeRoutes();
routes.MapRoute(
"Default", // Route name
"{controller}/{action}/{id}", // URL with parameters
new { controller = "Home", action = "about", id = UrlParameter.Optional } // Parameter defaults
);
}
}
Attribute Routing Example
A route attribute is defined on top of an action method. The following is the example of a Route Attribute in which routing is defined where the action method is defined.
In the following example, I am defining the route attribute on top of the action method
public class HomeController : Controller
{
//URL: /Mvctest
[Route(“Mvctest”)]
public ActionResult Index()
ViewBag.Message = "Welcome to ASP.NET MVC!";
return View();
}}
Attribute Routing with Optional Parameter
We can also define an optional parameter in the URL pattern by defining a question mark (“?") to the route parameter. We can also define the default value by using parameter=value.
public class HomeController : Controller
{
// Optional URI Parameter
// URL: /Mvctest/
// URL: /Mvctest/0023654
[Route(“Mvctest /{ customerName ?}”)]
public ActionResult OtherTest(string customerName)
ViewBag.Message = "Welcome to ASP.NET MVC!";
return View();
}
// Optional URI Parameter with default value
// URL: /Mvctest/
// URL: /Mvctest/0023654
[Route(“Mvctest /{ customerName =0036952}”)]
public ActionResult OtherTest(string customerName)
{
ViewBag.Message = "Welcome to ASP.NET MVC!";
return View();
}
}
Route Prefixes
We can also set a common prefix for the entire controller (all action methods within the controller) using the “RoutePrefix” attribute.
Example
[RoutePrefix(“Mvctest”)]
public class HomeController : Controller
{
// URL: /Mvctest/
[Route]
public ActionResult Index()
{
ViewBag.Message = "Welcome to ASP.NET MVC!";
return View();
}
// Optional URI Parameter
// URL: /Mvctest/
// URL: /Mvctest/0023654
[Route(“{ customerName }”)]
public ActionResult OtherTest(string customerName)
{
ViewBag.Message = "Welcome to ASP.NET MVC!";
return View();
}
}
When we use a tide (~) sign with the Route attribute, it will override the route prefix.
Example
[RoutePrefix(“Mvctest”)]
public class HomeController : Controller
{
// URL: /NewMvctest/
[Route(“~/NewMVCTest”)]
public ActionResult Index()
{
ViewBag.Message = "Welcome to ASP.NET MVC!";
return View();
}
}
Defining Default Route using Route Attribute
We can also define a Route attribute on top of the controller, to capture the default action method as the parameter.
Example
[RoutePrefix(“Mvctest”)]
[Route(“action=index”)]
public class HomeController : Controller
{
// URL: /Mvctest/
public ActionResult Index()
{
ViewBag.Message = "Welcome to ASP.NET MVC!";
return View();
}
// URL: /Mvctest/NewMethod
public ActionResult NewMethod()
{
ViewBag.Message = "Welcome to ASP.NET MVC!";
return View();
}
}
Defining Route name
We can also define a name of the route to allow easy URI generation.
Example
[Route(“Mvctest”, Name = "myTestURL")]
public ActionResult Index()
{
ViewBag.Message = "Welcome to ASP.NET MVC!";
return View();
}
We can generate URI using Url.RouteUrl method.
<a href="@Url.RouteUrl("mainmenu")">Test URI</a>
Defining Area
We can define the "Area" name from the controller that belongs to the using RouteArea attribute. If we define the “RouteArea” attribute on top of the controller, we can remove the AreaRegistration class from global.asax.
[RouteArea(“Test”)]
[RoutePrefix(“Mvctest”)]
[Route(“action=index”)]
public class HomeController : Controller
{
// URL: /Test/Mvctest/
public ActionResult Index()
{
ViewBag.Message = "Welcome to ASP.NET MVC!";
return View();
}
}
Attribute Routing gives us more control over the URIs in our MVC web application. The earlier way of routing (convention-based routing) is fully supported by this version of MVC. We can also use both type of routing in the same project.
Attribute Routing with Optional Parameter