
January 14, 2022 07:48 by
Peter
In this article, you will learn how to remove or customize View Engines not being used by an application.
If you are not using any view engine like ASPX View Engine, it is better to remove it to improve the performance, it is one of the many MVC performance tuning tips.
You might be wondering how it will improve the performance. Let's prove it by creating a new action method in a Home Controller, don't add a view for this action method now.
public ActionResult Foo()
{
return View();
}
Now, run the application and try navigating to "http://localhost:1212/Home/Foo". Here is what I received:
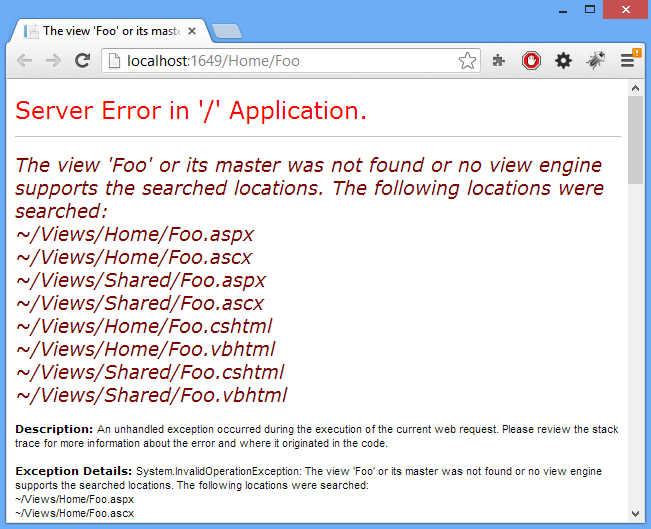
In the image above you can see how the MVC runtime is looking for an ASPX View Engine first and then the other view engines, which is still a default behavior.
You can control it from the Global.asax file by taking advantage of ViewEngines.Engines.Clear().
protected void Application_Start()
{
ViewEngines.Engines.Clear();
ViewEngines.Engines.Add(new RazorViewEngine());
AreaRegistration.RegisterAllAreas();
WebApiConfig.Register(GlobalConfiguration.Configuration);
FilterConfig.RegisterGlobalFilters(GlobalFilters.Filters);
RouteConfig.RegisterRoutes(RouteTable.Routes);
BundleConfig.RegisterBundles(BundleTable.Bundles);
AuthConfig.RegisterAuth();
}
I highlighted the newly added code above. Now, run the application; you will see the following:
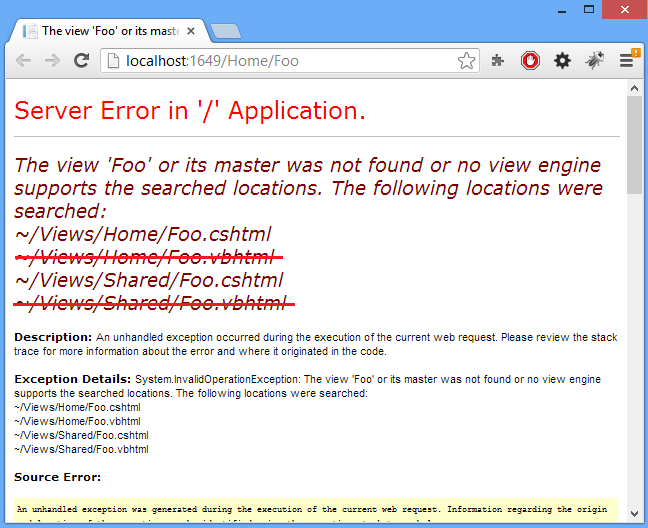
You will see that the MVC runtime successfully removed the ASPX View Engine but it is still looking for VBHTML Views.
You can also control it by customizing Razor View Engine to use only C# languages, by making some changes here:
ViewEngines.Engines.Add(new RazorViewEngine());
I highlighted the portion of code. This is actually calling the RazorViewEngine. RazorViewEngine() method internally that has support for both languages (C# and VB) by default. So, we need to override the default functionality by adding a class by the name "CustomRazorViewEngine" that inherits RazorViewEngine. The best place to add this class file is in the App_Start folder.
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
using System.Web.Mvc;
namespace MvcApplication1.App_Start
{
public class CustomRazorViewEngine : RazorViewEngine
{
public CustomRazorViewEngine()
{
base.AreaViewLocationFormats = new string[] {
"~/Areas/{2}/Views/{1}/{0}.cshtml",
"~/Areas/{2}/Views/Shared/{0}.cshtml"
};
base.AreaMasterLocationFormats = new string[] {
"~/Areas/{2}/Views/{1}/{0}.cshtml",
"~/Areas/{2}/Views/Shared/{0}.cshtml"
};
base.AreaPartialViewLocationFormats = new string[] {
"~/Areas/{2}/Views/{1}/{0}.cshtml",
"~/Areas/{2}/Views/Shared/{0}.cshtml"
};
base.ViewLocationFormats = new string[] {
"~/Views/{1}/{0}.cshtml",
"~/Views/Shared/{0}.cshtml"
};
base.PartialViewLocationFormats = new string[] {
"~/Views/{1}/{0}.cshtml",
"~/Views/Shared/{0}.cshtml"
};
base.MasterLocationFormats = new string[] {
"~/Views/{1}/{0}.cshtml",
"~/Views/Shared/{0}.cshtml"
};
}
}
}
Remember to use the System.Web.Mvc namespace. Now, in the Global.asax file add the following code:
protected void Application_Start()
{
ViewEngines.Engines.Clear();
//ViewEngines.Engines.Add(new RazorViewEngine());
ViewEngines.Engines.Add(new CustomRazorViewEngine());
AreaRegistration.RegisterAllAreas();
WebApiConfig.Register(GlobalConfiguration.Configuration);
FilterConfig.RegisterGlobalFilters(GlobalFilters.Filters);
RouteConfig.RegisterRoutes(RouteTable.Routes);
BundleConfig.RegisterBundles(BundleTable.Bundles);
AuthConfig.RegisterAuth();
}
Remember to use the MvcApplication1.App_Start namespace to bring the class file created above into the Global.asax scope. Now, run the application, you will see the following output:
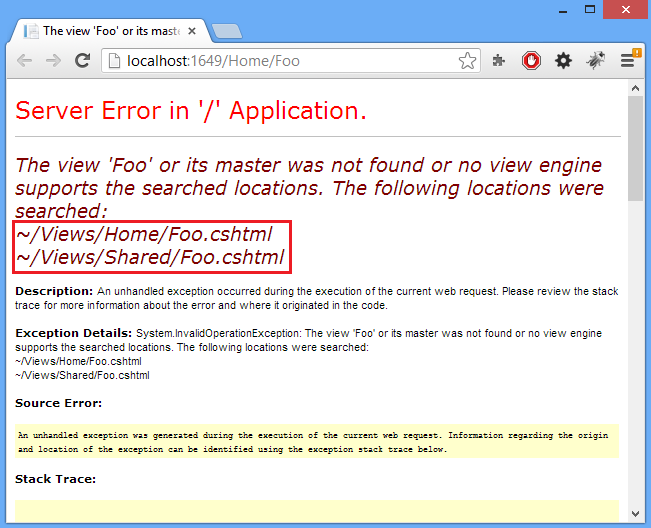