
August 4, 2023 07:40 by
Peter
In this tutorial, we will look at how to create a dynamic form with multiselect checkboxes in an ASP.NET MVC application. We'll concentrate on how to use jQuery AJAX to handle form submission, retrieve the selected checkboxes, and store the data to the database. The code provided offers a detailed example of how to develop a user-friendly student data management system that allows each student to select several languages.
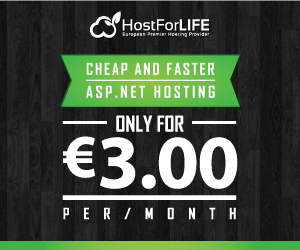
You should have a basic understanding of ASP.NET MVC, C#, jQuery, and Entity Framework before going.
How do you make a Multiselect Checkbox Form?
In the "Index.chtml" file, we have designed a form to collect student data, including a section to select multiple languages for each student using checkboxes.
<!-- Index.chtml -->
<!-- ... (existing code) ... -->
<div class="text-light text-center my-3">
<div class="d-flex justify-content-center">
<h5 class="text-warning">Languages:</h5>
<div id="languagesSection">
@for (int i = 0; i < Model.Languages.Count; i++)
{
<label>@Model.Languages[i].LanguageName</label>
@Html.CheckBoxFor(model => model.Languages[i].IsChecked)
@Html.HiddenFor(model => model.Languages[i].ID)
@Html.HiddenFor(model => model.Languages[i].LanguageName)
}
</div>
</div>
</div>
<!-- ... (existing code) ... -->
Implementing AJAX for Adding Data to Database
In the JavaScript section, we've implemented an AJAX function to handle the form submission and add student data to the database.
// AJAX for Adding Data to Database
$("#SumbitButton").click(function () {
var selected = []; // initialize array
$('div#languagesSection2 input[type=checkbox]').each(function () {
if ($(this).is(":checked")) {
selected.push($(this).attr("id"));
}
});
$("#Languages").val(selected);
var studentData = $("#studentInputForm").serialize();
if (!$('#studentInputForm').valid()) {
return false;
}
$.ajax({
url: "/Home/SaveStudent",
type: "POST",
data: studentData,
success: function (data) {
$('#studentInputForm').find(':input').val('');
$('input[type=checkbox]').prop('checked', false);
},
error: function (errormessage) {
console.log("error message");
}
});
});
Handling the AJAX Request in the Controller
In the "HomeController," we've added two action methods, one for saving student data and the other for handling the edit functionality.
public class HomeController : Controller
{
OperationRepositery repo = null;
public HomeController()
{
repo = new OperationRepositery();
}
public ActionResult Index()
{
StudentModel model = new StudentModel();
model.Languages = db.LanguagesTable.Select(lang => new LanguagesModel
{
ID = lang.ID,
LanguageName = lang.LanguagesName,
}).ToList();
return View(model);
}
// Action method for adding student data
public ActionResult SaveStudent(StudentModel model)
{
var result = repo.AddData(model);
return Json(new { result = true }, JsonRequestBehavior.AllowGet);
}
// Action method for handling the edit functionality
public ActionResult EditStudent(int ID)
{
var result = repo.EditFunction(ID);
var languageIdList = result.LanguageIds.Split(',').Select(x => Convert.ToInt32(x)).ToList();
result.Languages = db.LanguagesTable.Select(x => new LanguagesModel
{
ID = x.ID,
LanguageName = x.LanguagesName,
IsChecked = languageIdList.Contains(x.ID)
}).ToList();
return View(result);
}
}
Happy coding!