When paired with the Clean Architecture pattern, ASP.NET Core MVC provides a solid framework for building online applications that can result in controllable and extendable solutions. In this article, we will look at how to organize an ASP.NET Core MVC project utilizing Clean Architecture principles, using C# code samples.
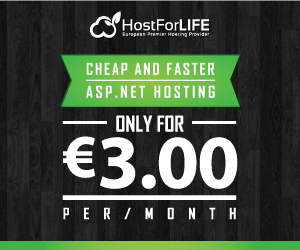
What is the Clean Architecture Concept?
Clean Architecture is a software design philosophy that emphasizes concern separation and maintainability by layering the codebase. Typically, these layers include.
Layer of User Interface
The presentation elements are placed here. It includes controllers, views, and view models in the context of ASP.NET Core MVC.
Layer of Application
This layer contains the application's business logic. It is in charge of managing user queries, data processing, and interfacing with the domain layer.
The Domain Layer
This layer is the application's heart, defining the fundamental business entities, rules, and domain-specific logic. It must be decoupled from any infrastructure or application-specific code.
Layer of Infrastructure
This layer handles external concerns like data access, external services, and infrastructure-related code. It should be kept separate from the other layers.
Establishing a Clean Architecture Initiative
Let's get started by making a new ASP.NET Core MVC project with Clean Architecture in mind.
Step One: Make a New ASP.NET Core MVC Project
To start a new ASP.NET Core MVC project, we can use either the dotnet command-line tool or Visual Studio. Ascertain that the ASP.NET Core SDK is installed.
dotnet new mvc -n ZRCleanArchitectureApp
Step 2: Creating a Project Structure
Separate our project into distinct folders for each layer to better organize it.
ZRCleanArchitectureApp.Web
This folder comprises controllers, views, and view models and represents the presentation layer.
ZRCleanArchitectureApp.Application
We define application services and business logic in this subdirectory.
ZRCleanArchitectureApp.Domain
This folder contains the definitions of our domain entities and business rules.
ZRCleanArchitectureApp.Infrastructure
This section handles data access, external services, and infrastructure-related code.
Step 3: Put Clean Architecture into Practice
Domain Entities Definition
// ZRCleanArchitectureApp.Domain/Entities/Product.cs
public class Product
{
public int Id { get; set; }
public string Name { get; set; }
public decimal Price { get; set; }
}
Implementing Application Services
// ZRCleanArchitectureApp.Application/Services/ProductService.cs
public class ProductService
{
private readonly IProductRepository _productRepository;
public ProductService(IProductRepository productRepository)
{
_productRepository = productRepository;
}
public async Task<IEnumerable<Product>> GetAllProductsAsync()
{
return await _productRepository.GetAllAsync();
}
// Add other business logic methods here
}
Creating Controllers
// ZRCleanArchitectureApp.Web/Controllers/ProductController.cs
public class ProductController : Controller
{
private readonly ProductService _productService;
public ProductController(ProductService productService)
{
_productService = productService;
}
public async Task<IActionResult> Index()
{
var products = await _productService.GetAllProductsAsync();
return View(products);
}
// Add other action methods
}
Configuring Dependency Injection
In the Program.cs file, configure dependency injection for our services and repositories.
builder.Services.AddScoped<ProductService>();
builder.Services.AddScoped<IProductRepository, ProductRepository>();
ASP.NET Core MVC Clean Architecture provides a disciplined approach to designing maintainable, scalable, and easily testable web applications. We may focus on building clean, modular code by dividing concerns into discrete levels.
We have barely scraped the surface of Clean Architecture in this post. We may improve our project by incorporating validation, authentication, and authorization systems, as well as unit tests to check the accuracy of our code.
Keep in mind that Clean Architecture is a suggestion, not a hard and fast rule. Adapt it to our project's unique requirements and complexities, and strive for simplicity and maintainability in our codebase at all times.