This article is an overview of the use of ActionResult in ASP.Net Core MVC. ASP.NET Core MVC has different types of Action Results. Each action result returns a different format of the output. As a programmer, we need to use different action results to get the expected output.
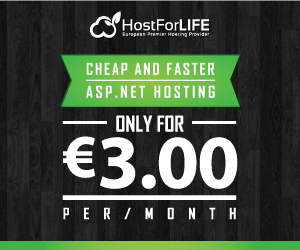
What is Action Method in ASP.NET Core MVC?
Actions are the methods in controller class which are responsible for returning the view or Json data. Action will mainly have return type “ActionResult” and it will be invoked from method InvokeAction called by controller. All the public methods inside a controller which respond to the URL are known as Action Methods. When creating an Action Method we must follow these rules. I have divided all the action methods into the following categories:
- ActionResult
- RedirectActionResult
- FileResult
- Security
Miscellaneous Action Results
Action Method
|
Description
|
IActionResult
|
Defines a contract that represents the result of an action method.
|
ActionResult
|
A default implementation of IActionResult.
|
ContentResult
|
Represents a text result.
|
EmptyResult
|
Represents an ActionResult that when executed will do nothing.
|
JsonResult
|
An action result which formats the given object as JSON.
|
PartialViewResult
|
Represents an ActionResult that renders a partial view to the response.
|
ViewResult
|
Represents an ActionResult that renders a view to the response.
|
ViewComponentResult
|
An IActionResult which renders a view component to the response.
|
ActionResult
The IActionResult return type is appropriate when multiple ActionResult return types are possible in an action. IActionResult and ActionResult work as a container for other action results, in that IActionResult is an interface and ActionResult is an abstract class that other action results inherit from.
public IActionResult Index()
{
return View();
}
ActionResult
ActionResult is the base class of all the result type action method.
public ActionResult About()
{
return View();
}
ContentResult
ContentResult represents a text result. The default return type of a ContentResult is string, but it’s not limited to string. We can return any type of response by specifying a MIME type, in the code.
public ContentResult ContentResult()
{
return Content("I am ContentResult");
}
EmptyResult
EmptyResult represents an ActionResult that when executed will do nothing. We can use it when we want to return empty result.
public EmptyResult EmptyResult()
return new EmptyResult();
JsonResult
JsonResult formats the given object as JSON. JsonResult is use to return JSON-formatted data, it returns JSON regardless of what format is requested through Accept header. There is no content negotiation when we use JsonResult.
public JsonResult JsonResult()
{
var name = "Farhan Ahmed";
return Json(new { data=name});
}
PartialViewResult
PartialViewResult represents an ActionResult that renders a partial view to the response. PartialViews are essential when it comes to loading a part of page through AJAX, they return raw rendered HTML.
public PartialViewResult PartialViewResult()
{
return PartialView("_PartialView");
}
ViewResult
ViewResult represents an ActionResult that renders a view to the response. It is used to render a view to response, we use it when we want to render a simple .cshtml view.
public ViewResult ViewResult()
{
return View("About","Home");
}
ViewComponentResult
ViewComponentResult is an IActionResult which renders a view component to the response. We use view component by calling Component.InvokeAsync in the view. We can use it to return HTML form a view component. If we want to reuse our business logic or refresh the HTML parts of the page that are loaded with view component we can do that using view component.
public ViewComponent ViewComponent()
{
return ViewComponent();
}
Action results from previous version of ASP.NET MVC that are either renamed or deleted.
- JavaScriptResult - This action result does not exist anymore we can use ContentResult.
- FilePathResult - We can use VirtualFileResult or PhysicalFileResult instead of FilePathResult
- HttpNotFoundResult - We can use NotFoundResult instead HttpNotFoundResult
- HttpStatusCodeResult - We can use StatusCodeResult instead HttpStatusCodeResult
- HttpUnauthorizedResult - We can use UnauthorizedResult instead HttpUnauthorizedResult