
January 15, 2021 08:56 by
Peter
This article overviews redirect action results in ASP.NET Core MVC. We will lean all redirect action results step by step with examples.
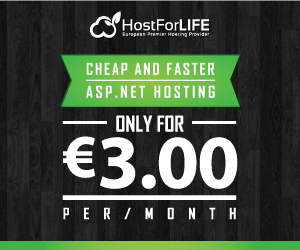
There are four types of redirect action results in ASP.Net Core MVC. Each one can either return normal redirect or permanent. The return method related to the permanent one is suffixed with the Permanent keyword. You can also return these results with their Permanent property set to true. These action results are:
RedirectResult
RedirectToActionResult
RedirectToRouteResult
LocalRedirectResult
RedirectResult
RedirectResult is an ActionResult that returns a Found (302), Moved Permanently (301), Temporary Redirect (307), or Permanent Redirect (308) response with a Location header to the supplied URL. It will redirect us to the provided URL, it doesn’t matter if the URL is relative or absolute.
public RedirectResult MyProfile()
{
return Redirect("https://www.c-sharpcorner.com/members/farhan-ahmed24");
}
public RedirectResult Profile()
{
return RedirectPermanent("https://www.c-sharpcorner.com/members/farhan-ahmed24");
}
If we call the RedirectPermanent method, it redirect us permanently. Also as I explained in previous section we don’t need to use these methods to redirect permanently or temporarily, we can just new up an instance of RedirectResult with its Permanent property set to true or false and return that instead.
return new RedirectResult("/") { Permanent = true};
RedirectToActionResult
RedirectToActionResult is an ActionResult that returns a Found (302), Moved Permanently (301), Temporary Redirect (307), or Permanent Redirect (308) response with a Location header. It targets a controller action, taking in action name, controller name, and route value.
public RedirectToActionResult EmployeeList()
{
return RedirectToAction("Index", "Employees");
}
RedirectToRouteResult
RedirectToRouteResult is an ActionResult that returns a Found (302), Moved Permanently (301), Temporary Redirect (307), or Permanent Redirect (308) response with a Location header. Targets a registered route. It should be used when we want to redirect to a route. It takes a route name, route value and redirect us to that route with the route values provided.
public RedirectToRouteResult DepartmentList()
{
return RedirectToRoute(new { action = "Index", controller = "Departments", area="" });
}
LocalRedirectResult
LocalRedirectResult is an ActionResult that returns a Found (302), Moved Permanently (301), Temporary Redirect (307), or Permanent Redirect (308) response with a Location header to the supplied local URL. We should use LocalRedirectResult if we want to make sure that the redirects occur in some context that are local to our site. This action result type takes a string for URL needed for redirect, and a bool flag to tell it if it’s permanent. Under the hood, it checks the URL with the Url.IsLocalUrl("URL") method to see if it’s local. If it redirects us to the address, but if it wasn’t, it will throw an InvalidOperationException. You cannot pass a local URL with an absolute address like this, http://localhost:52513/Home/Index, you’ll get an exception. That’s because the IsLocalUrl method considers a URL like this to not be local, so you must always pass a relative URL in.
public LocalRedirectResult LocalRedirect()
{
return LocalRedirect("/Home/Index");
}
public LocalRedirectResult LocalRedirectActionResult()
{
var IsHomeIndexLocal = Url.IsLocalUrl("/Home/Index");
var isRootLocal = Url.IsLocalUrl("/");
var isAbsoluteUrlLocal = Url.IsLocalUrl("http://localhost: 52513/Home/Index");
return LocalRedirect("/Home/Index");
}
Step 1
Open Visual Studio 2019 and select the ASP.NET Core Web Application template and click Next.
Step 2
Name the project FileResultActionsCoreMvc_Demo and click Create.
Step 3
Select Web Application (Model-View-Controller), and then select Create. Visual Studio used the default template for the MVC project you just created.
Step 4
In Solution Explorer, right-click the wwwroot folder. Select Add > New Folder. Name the folder Files. Add some files to work with them.
Complete controller code
using System;
using System.Collections.Generic;
using System.Diagnostics;
using System.Linq;
using System.Threading.Tasks;
using Microsoft.AspNetCore.Mvc;
using Microsoft.Extensions.Logging;
using RedirectResultActionsCoreMvc_Demo.Models;
namespace RedirectResultActionsCoreMvc_Demo.Controllers
{
public class HomeController : Controller
{
public IActionResult Index()
{
return View();
}
public RedirectResult MyProfile()
{
return Redirect("https://www.c-sharpcorner.com/members/farhan-ahmed24");
}
public RedirectResult Profile()
{
return RedirectPermanent("https://www.c-sharpcorner.com/members/farhan-ahmed24");
}
public RedirectToActionResult EmployeeList()
{
return RedirectToAction("Index", "Employees");
}
public RedirectToRouteResult DepartmentList()
{
return RedirectToRoute(new { action = "Index", controller = "Departments", area="" });
}
public LocalRedirectResult LocalRedirect()
{
return LocalRedirect("/Home/Index");
}
}
}
Step 5
Open Index view which is in the views folder under the Home folder. Add the below code in Index view.
Index View
@{
ViewData["Title"] = "Home Page";
}
<h3 class="text-uppercase">RedirectResult Action in core mvc</h3>
<ul class="list-group list-group-horizontal">
<li class="list-group-item"><a asp-action="MyProfile" asp-controller="Home" target="_blank">Redirect Result</a></li>
<li class="list-group-item"><a asp-action="EmployeeList" asp-controller="Home" target="_blank">RedirectToActionResult</a></li>
<li class="list-group-item"><a asp-action="DepartmentList" asp-controller="Home" target="_blank">RedirectToRouteResult</a></li>
<li class="list-group-item"><a asp-action="LocalRedirect" asp-controller="Home" target="_blank">LocalRedirectResultt</a></li>
</ul>
Step 6
Build and run your Project, ctrl+F5
