
June 27, 2024 08:03 by
Peter
Performance optimization for ASP.NET MVC (Model-View-Controller) applications use a range of techniques in an effort to improve the efficiency, responsiveness, and speed of applications created using this framework. The following are some key areas and methods for optimizing the performance of ASP.NET MVC applications.
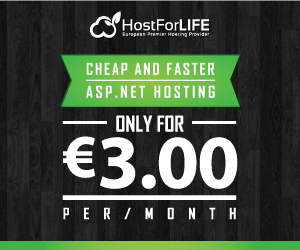
1. Efficient data access
Use Entity Framework wisely: Optimize queries, use lazy loading carefully, and prefer compiled queries for frequently used data access paths.
Stored Procedures: Use stored procedures for complex and frequently run queries to reduce the overhead of query parsing and execution planning.
Caching: Implement caching strategies like output caching, data caching, and distributed caching (e.g., using Redis) to reduce database load.
2. Optimizing server-side code
Async/Await: Use asynchronous programming (async/await) to handle I/O-bound operations without blocking threads.
Minimize ViewState: Reduce the use of ViewState to decrease the payload size.
Bundling and Minification: Combine and minify CSS and JavaScript files to reduce the number of HTTP requests and the size of requested resources.
Compression: Enable GZIP compression in IIS to compress the response data sent to the client, reducing the response size.
3. Efficient use of resources
Pooled Connections: Use connection pooling to reuse database connections.
Memory Management: Properly manage memory usage to avoid excessive garbage collection and memory leaks.
Thread Pooling: Utilize thread pooling to manage a pool of worker threads, which can be reused for executing tasks, reducing the overhead of thread creation.
4. Front-end performance
Lazy Loading: Implement lazy loading for images and other resources to load them only when they are in the viewport.
Content Delivery Network (CDN): Use CDNs to serve static resources like images, CSS, and JavaScript files from locations closer to the user, reducing latency.
Browser Caching: Set appropriate caching headers to enable browsers to cache static resources.
5. Optimizing Queries and Data Access
Indexing: Properly index database tables to improve query performance.
Pagination: Implement pagination for data-heavy views to load only a subset of data at a time.
Query Optimization: Analyze and optimize LINQ queries to reduce execution time.
6. Application Monitoring and Profiling
Application Insights: Use tools like Azure Application Insights for monitoring and diagnostics to identify performance bottlenecks.
Profiling Tools: Use profiling tools to analyze the performance of your application and identify hotspots.
7. Configuration and Deployment
Configuration Settings: Tune application settings such as session state management, request timeouts, and garbage collection modes.
Scalability: Design the application to be scalable, allowing it to handle increased load by adding more resources (horizontal scaling) or optimizing resource usage (vertical scaling).
8. Load Balancing
Load Balancers: Use load balancers to distribute incoming traffic across multiple servers, ensuring no single server is overwhelmed.
Session State Management: Ensure the session state is managed in a distributed manner (e.g., using a distributed cache) to support load balancing.
Example implementation
Here’s an example of how to implement output caching in an ASP.NET MVC controller.
using System.Web.Mvc;
public class HomeController : Controller
{
[OutputCache(Duration = 60, VaryByParam = "none")]
public ActionResult Index()
{
return View();
}
}
In this example, the OutputCache attribute is applied to the Index action method, caching the output for 60 seconds.
Conclusion
By implementing these strategies, you can significantly improve the performance of your ASP.NET MVC application. Each application is unique, so it's important to profile and monitor your specific application to identify the most effective optimization techniques.