
November 14, 2023 06:11 by
Peter
There are numerous Payment Gateways on the market that provide secure and simple setup. Stripe is among them. Stripe integration consists of numerous phases. In general, there are two approaches.
- Client Side: You may use Stripe.js to load the Stripe UI and enter credit card information to make the payment.
- If you already have a fully functional Checkout page and everything is in place, you may utilize the library (DLL) to process the payment.
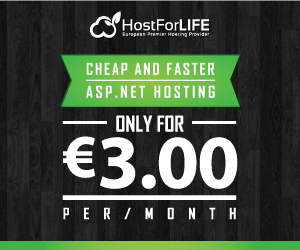
Today, we'll look at Stripe's server-side implementation.
Stripe Payment Gateway can be readily integrated if you follow each step.
Step 1: Fill out the registration form at https://dashboard.stripe.com/register with your email, name, and password.
Step 2: Use Install-Package Stripe.net to add the Stripe library to your Visual Project. Stripe.net.dll will be downloaded and included as a reference to your project.
Step 3: Add the Stripe.Infrastructure; Namespace to the Class where you wish to create the payment gateway.
Step 4: To implement, you must experiment with various classes. To make the payment, please complete each step.
Step 5: You'll need KEY - "Publishable key" - to connect to Stripe. It is available at https://dashboard.stripe.com/account/apikeys.

Step 6. Set the API key with the below function
Stripe.StripeConfiguration.SetApiKey(“pk_test_FyPZYPyqf8jU6IdG2DONgudS”);
Step 7: To generate a Token, create an Object of a Credit Card. That Token will be assigned to the Customer object at the time the Customer is created.
//Create Card Object to create Token
Stripe.CreditCardOptions card = new Stripe.CreditCardOptions();
card.Name = tParams.CardOwnerFirstName + " " + tParams.CardOwnerLastName;
card.Number = tParams.CardNumber;
card.ExpYear = tParams.ExpirationYear;
card.ExpMonth = tParams.ExpirationMonth;
card.Cvc = tParams.CVV2;
//Assign Card to Token Object and create Token
Stripe.TokenCreateOptions token = new Stripe.TokenCreateOptions();
token.Card = card;
Stripe.TokenService serviceToken = new Stripe.TokenService();
Stripe.Token newToken = serviceToken.Create(token);
Step 8: Assign TokenID to the Customer Object so that a card is created and linked to the Customer when the customer is created.
//Create Customer Object and Register it on StripeStripe.CustomerCreateOptions myCustomer = new Stripe.CustomerCreateOptions();myCustomer.Email = tParams.Buyer_Email;myCustomer.SourceToken = newToken.Id;var customerService = new Stripe.CustomerService();Stripe.Customer stripeCustomer = customerService.Create(myCustomer);
Step 9: Make a Charge Object. The charge object is the actual object that will perform the payment.
//Create Charge Object with details of Charge
var options = new Stripe.ChargeCreateOptions {
Amount = Convert.ToInt32(tParams.Amount),
Currency = tParams.CurrencyId == 1 ? "ILS" : "USD",
ReceiptEmail = tParams.Buyer_Email,
CustomerId = stripeCustomer.Id,
Description = Convert.ToString(tParams.TransactionId), //Optional
};
//and Create Method of this object is doing the payment execution.
var service = new Stripe.ChargeService();
Stripe.Charge charge = service.Create(options); // This will do the Payment
Step ten: Charge.The status will be returned.
Step 11: Go to https://dashboard.stripe.com/test/customers and look for Created Customer.

And Payment using this link - https://dashboard.stripe.com/test/payments.
