Cross-site Scripting, or PDF XSS, is another name for PDF Injection, which is a potentially dangerous security flaw. In an MVC (Model-View-Controller) application, it is imperative to include appropriate validation and sanitation techniques to prevent such difficulties during PDF upload and viewing. The sample code that shows how to handle PDF uploads safely and stop PDF Injection from causing Cross-site Scripting in an MVC environment is provided below.
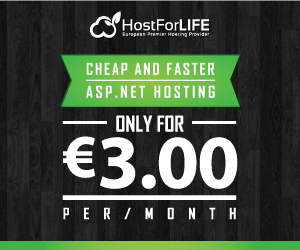
Assuming you are working with a well-known MVC framework such as ASP.NET MVC, the following is a C# example that is simplified:
PDF Upload Validation: Implement server-side validation to ensure that only legitimate PDF files are accepted throughout the upload process.
[HttpPost]
public ActionResult UploadPdf(HttpPostedFileBase pdfFile)
{
if (pdfFile != null && pdfFile.ContentLength > 0)
{
// Check if the uploaded file is a PDF
if (pdfFile.ContentType != "application/pdf" || !pdfFile.FileName.EndsWith(".pdf"))
{
ModelState.AddModelError("pdfFile", "Only PDF files are allowed.");
return View("Upload");
}
// Process the PDF file
// ...
}
return RedirectToAction("Index");
}
Viewing a PDF: Use secure libraries that don't run any embedded JavaScript while rendering the PDF for viewing. Don't forget to sanitize the PDF's contents.
public ActionResult ViewPdf(int fileId)
{
// Fetch the PDF file from the database or file system based on the fileId
var pdfContent = GetPdfContent(fileId);
// Sanitize the PDF content to prevent XSS
var sanitizedPdfContent = SanitizePdfContent(pdfContent);
// Render the sanitized PDF content
return File(sanitizedPdfContent, "application/pdf");
}
Sanitizing PDF Content: To stop JavaScript from running, implement a function that sanitizes the PDF content.
private byte[] SanitizePdfContent(byte[] pdfContent)
{
// Implement PDF content sanitization logic here
// Check that the PDF content does not contain malicious scripts
// Example: Using a library to remove JavaScript from the PDF content
// var sanitizedContent = PdfSanitizationLibrary.Sanitize(pdfContent);
// Return the sanitized PDF content
// return sanitizedContent;
// For illustration purposes, let's assume no sanitization for simplicity
return pdfContent;
}
This is a rudimentary example; in a real-world situation, you might want to make sure that the PDF processing library is secure against PDF Injection and utilize a separate library. To reduce the danger of cross-site scripting (XSS) attacks, think about adding Content Security Policy (CSP) headers to your online application.
To take advantage of security updates and enhancements, make sure your libraries and frameworks are up to date at all times.
To sum up, strengthening your web environment begins with giving your MVC application's security first priority when it comes to PDF uploads. Strong validation checks put in place during the upload process create a strong initial line of defense against efforts at harmful PDF Injection. Choosing safe and up-to-date PDF rendering libraries reduces the possibility of Cross-site Scripting (XSS) vulnerabilities by guaranteeing that the material shown to users is free of malicious scripts. By including content sanitization procedures, a thorough defense against potential security threats is provided, adding an additional layer of protection.
It's critical to remain proactive as the digital landscape changes constantly. It is essential to keep your application's dependencies, such as PDF processing libraries, up to date in order to take advantage of the most recent security improvements. Your development lifecycle should include regular penetration tests and security assessments as they can assist find and fix new vulnerabilities. Following these guidelines will make your MVC application more resilient and help your users have a safer and more reliable online experience.