ASP.NET MVC enables developers to link server-side models and user interface elements with ease in the dynamic world of web development. In this process, model binding is essential, and data annotations offer a potent toolkit for specifying metadata about the features of the model. This blog offers a thorough how-to for ASP.NET MVC newcomers, with the goal of demystifying the relationship between model binding and data annotations.
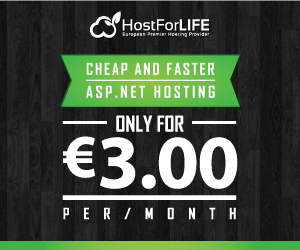
The Model-View-Controller architectural pattern, which is used by ASP.NET MVC, has views handle display while controllers handle user input and coordinate interactions between views and models. Models contain data and business logic. The process by which user input from a form or other sources is used to populate the model properties is known as model binding.
On the other side, characteristics added to model properties to provide metadata about how they should be handled during model binding, validation, and rendering are known as data annotations.
Model Binding Basics
Consider a simple scenario where you have a Person model.
public class Person
{
public string FirstName { get; set; }
public string LastName { get; set; }
public int Age { get; set; }
}
It may be desirable to connect user input to this model in a controller action.
[HttpPost]
public ActionResult SavePerson(Person person)
{
// Process the person object
// ...
}
Introducing Data Annotations
Now, let's enhance the Person model with data annotations to provide more information to the model binder.
public class Person
{
[Required(ErrorMessage = "First Name is required")]
[StringLength(50, ErrorMessage = "First Name should be less than 50 characters")]
public string FirstName { get; set; }
[Required(ErrorMessage = "Last Name is required")]
[StringLength(50, ErrorMessage = "Last Name should be less than 50 characters")]
public string LastName { get; set; }
[Range(1, 150, ErrorMessage = "Age should be between 1 and 150")]
public int Age { get; set; }
}
Important Data Annotations Employed
- Indicates that a certain attribute is necessary.
- StringLength: Indicates a string property's maximum length.
- Range: Indicates a numeric property's value range.
Making the Most of Data Annotations in Views
@using (Html.BeginForm("SavePerson", "YourController", FormMethod.Post))
{
@Html.LabelFor(model => model.FirstName)
@Html.TextBoxFor(model => model.FirstName)
@Html.ValidationMessageFor(model => model.FirstName)
// Repeat for LastName and Age properties
<input type="submit" value="Save" />
}
Important HTML Helpers Employed
- The label element for a model attribute is rendered by LabelFor.
- Renders a text input element for a model attribute using TextBoxFor.
- ValidationMessageFor: Validation error messages for a model property rendered by the renderer.
In-Progress Model Validation
The model binder automatically validates the user input when the form is submitted by using the data annotations. Error notifications appear next to the respective form fields if any validation fails.
HTML helpers can be used to render form components in a view. Annotations on data affect the generation and validation of these elements.
Data Annotations' Advantages in Model Binding
Consistency: Data annotations offer a declarative and consistent means of defining validation criteria, guaranteeing consistency throughout the program.
Readability: Developers can readily comprehend the limitations and specifications of each property since validation rules are incorporated directly into the model.
Reuse: Data annotations facilitate code reuse by allowing the same model to be applied with identical validation rules across contexts.
Validating form data and processing user input are made easier using ASP.NET MVC model binding and data annotations. Developers can define validation criteria succinctly within the model itself by utilizing properties like Required, StringLength, and Range. This method makes code more readable, encourages consistency, and makes it easier to maintain reliable and user-friendly online applications. Embrace the power of model binding and data annotations as you begin your ASP.NET MVC adventure to build applications that are robust and user-friendly.