I was inspired to produce an article about utilizing Dependency Injection in ASP.NET MVC5 after reading many ones about it in MVC and C#.
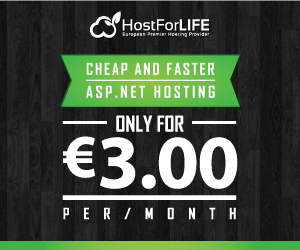
In MVC, Dependency Injection (DI)
One way to put "Inversion of Control" into practice is through dependency injection. According to the theory known as Inversion of Control (IoC), objects obtain the objects they require from an external source (such as an XML configuration file) rather than creating other objects that they depend on to do their tasks.
Let's now put the same into practice.
Create a new project for ASP.NET MVC.
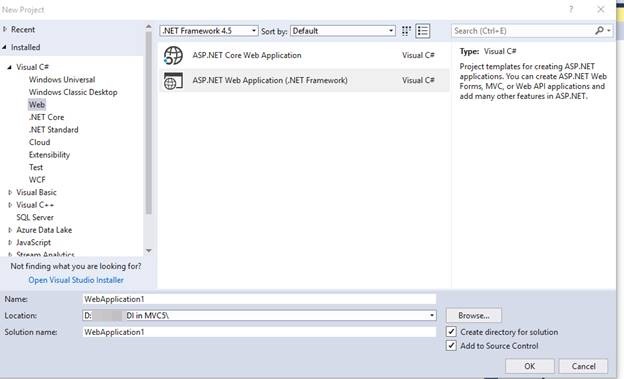
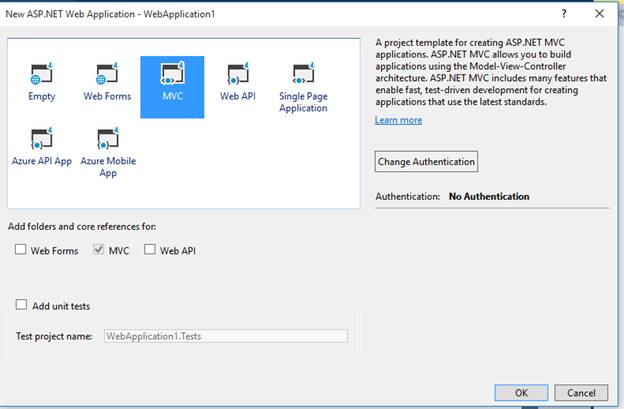
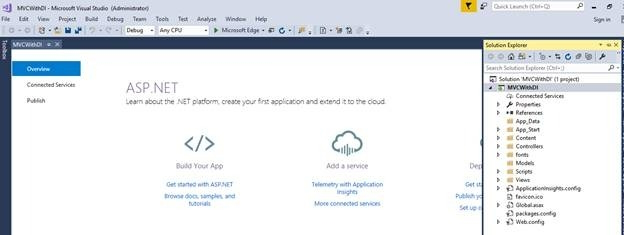
No, install the "Unity.Mvc5" Container using NuGet Package Manager, as shown below.
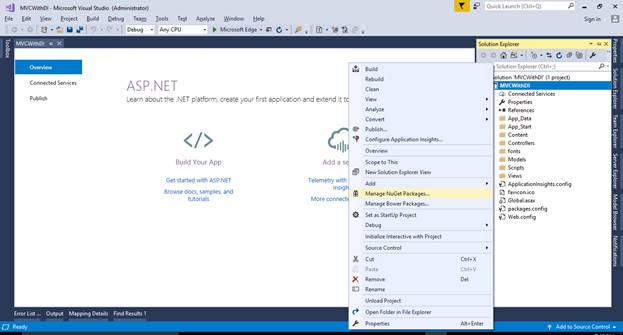
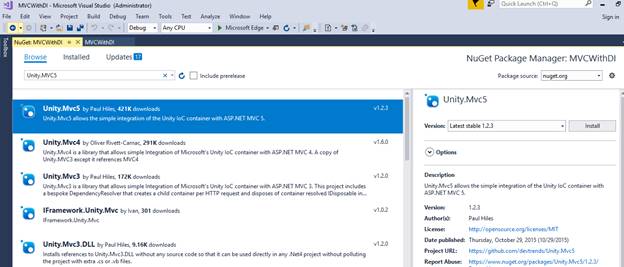
When it is installed successfully, you will find the following two references added to your project and a UnityConfig.cs class file in App-Start folder.
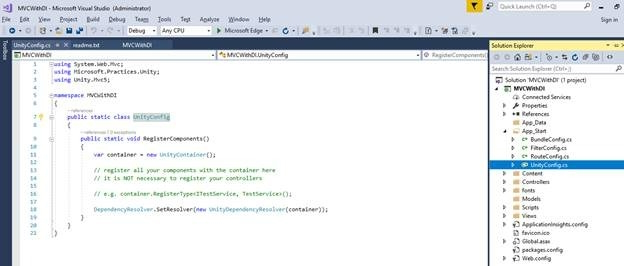
Now, let’s create the repository that will be accessed by the Controller.
- Add a folder named Repository.
- Add an interface IUserMasterRepository.
interface IUserMasterRepository
{
IEnumerable<UserMaster> GetAll();
UserMaster Get(int id);
UserMaster Add(UserMaster item);
bool Update(UserMaster item);
bool Delete(int id);
}
Now, add the repository which has your data access code.
public class UserMasterRepository : IUserMasterRepository
{
private List<UserMaster> users = new List<UserMaster>();
private int Id = 1;
public UserMasterRepository()
{
// Add products for the Demonstration
Add(new UserMaster { Name = "User1", EmailID = "[email protected]", MobileNo = "1234567890" });
Add(new UserMaster { Name = "User2", EmailID = "[email protected]", MobileNo = "1234567890" });
Add(new UserMaster { Name = "User3", EmailID = "[email protected]", MobileNo = "1234567890" });
}
public UserMaster Add(UserMaster item)
{
if (item == null)
{
throw new ArgumentNullException("item");
}
item.ID = Id++;
users.Add(item);
return item;
}
public bool Delete(int id)
{
users.RemoveAll(p => p.ID == id);
return true;
}
public UserMaster Get(int id)
{
return users.FirstOrDefault(x => x.ID == id);
}
public IEnumerable<UserMaster> GetAll()
{
return users;
}
public bool Update(UserMaster item)
{
if (item == null)
{
throw new ArgumentNullException("item");
}
int index = users.FindIndex(p => p.ID == item.ID);
if (index == -1)
{
return false;
}
users.RemoveAt(index);
users.Add(item);
return true;
}
}
Note. Here, we have used a repository. You can use services which will consume your Repository.
Now, register this repository to container in UnityConfig.cs.
public static void RegisterComponents()
{
var container = new UnityContainer();
container.RegisterType<IUserMasterRepository, UserMasterRepository>();
DependencyResolver.SetResolver(new UnityDependencyResolver(container));
}
Add UnityConfiguration in AppStart method of Global.asax
protected void Application_Start()
{
AreaRegistration.RegisterAllAreas();
FilterConfig.RegisterGlobalFilters(GlobalFilters.Filters);
RouteConfig.RegisterRoutes(RouteTable.Routes);
BundleConfig.RegisterBundles(BundleTable.Bundles);
UnityConfig.RegisterComponents();
}
Inject the Dependency in Controller.
Create UserController
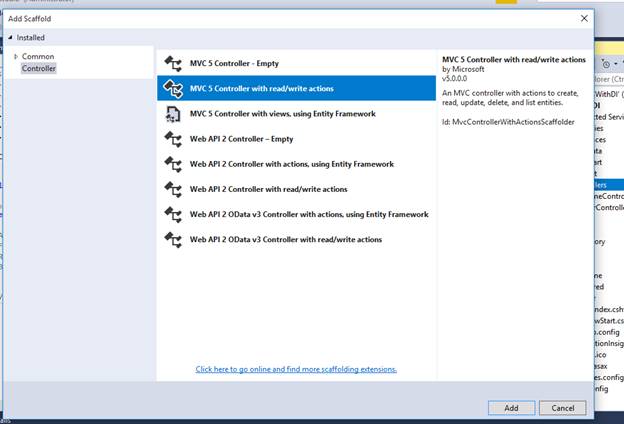
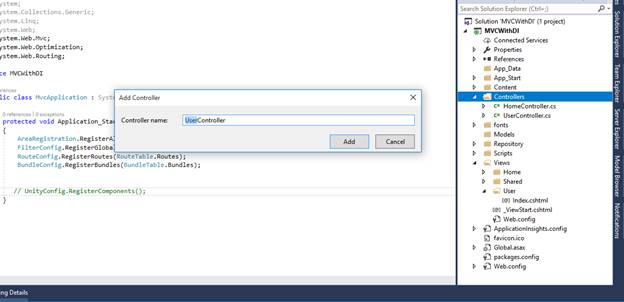
Now, in the below code, we have created a constructor of UserContoller, injected the UserMasterRepository, and accessed it in Index action.
public class UserController : Controller
{
readonly IUserMasterRepository userRepository;
public UserController(IUserMasterRepository repository)
{
this.userRepository = repository;
}
// GET: User
public ActionResult Index()
{
var data = userRepository.GetAll();
return View(data);
}
}
Add a View for the same.
Add User folder in Views folder.
Add Index View.
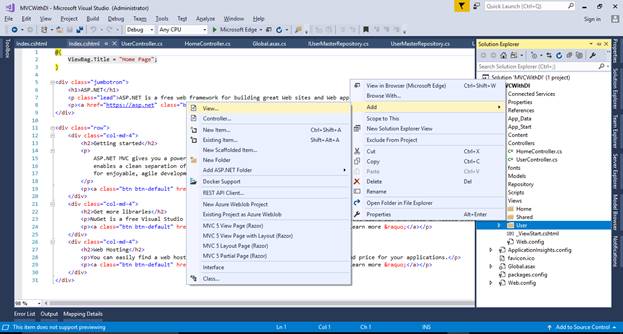
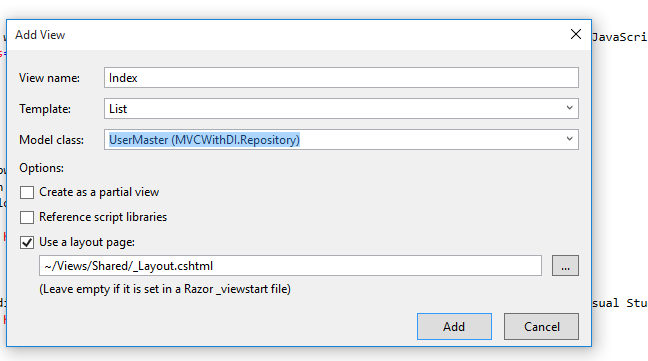
Below is the code which needs to be written in Index View file.
@model IEnumerable<MVCWithDI.Repository.UserMaster>
@{
ViewBag.Title = "Users";
Layout = "~/Views/Shared/_Layout.cshtml";
}
<h2>Index</h2>
<p>
@Html.ActionLink("Create New", "Create")
</p>
<table class="table">
<tr>
<th>
@Html.DisplayNameFor(model => model.Name)
</th>
<th>
@Html.DisplayNameFor(model => model.EmailID)
</th>
<th>
@Html.DisplayNameFor(model => model.MobileNo)
</th>
<th></th>
</tr>
@foreach (var item in Model) {
<tr>
<td>
@Html.DisplayFor(modelItem => item.Name)
</td>
<td>
@Html.DisplayFor(modelItem => item.EmailID)
</td>
<td>
@Html.DisplayFor(modelItem => item.MobileNo)
</td>
<td>
@Html.ActionLink("Edit", "Edit", new { id=item.ID }) |
@Html.ActionLink("Details", "Details", new { id=item.ID }) |
@Html.ActionLink("Delete", "Delete", new { id=item.ID })
</td>
</tr>
}
</table>
Now, run the project. Here is the output.
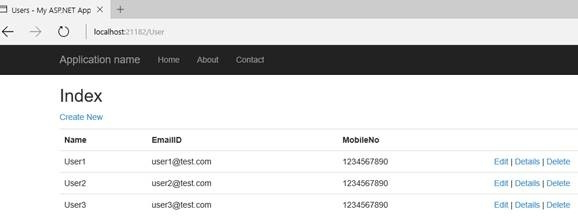