
April 2, 2024 07:32 by
Peter
In ASP.NET MVC, the ActionResult class plays a crucial part in the result that is sent to the client when an action method is invoked. It's important to know the different ActionResult types and when to use them when developing MVC applications that are both secure and effective. This article will examine the various types of ActionResult and provide usage examples.
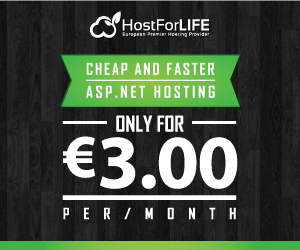
View Outcome
- Use this technique when producing an entire HTML view in response to a user request.
- typically used to display entire views or pages containing significant information.
- ideal for displaying intricate information representations or user interface components.
public ActionResult Index()
{
return View();
}
PartialViewResult
- Purpose- Including particular page portions by rendering partial views.
- Use Case- Perfect for containing reusable user interface elements such as sidebars, footers, headers, or widgets.Helps in maintaining modular and reusable code by separating UI components into smaller parts.
public ActionResult Sidebar()
{
return PartialView("_Sidebar");
}
RedirectResult
- Use when the client needs to be redirected to an alternative URL.
- frequently used following successful login/logout processes, form submissions, or the permanent move of a resource.
- helpful for handling URL changes or for putting HTTP redirects into place for SEO reasons.
public ActionResult RedirectCsharpcorner()
{
return Redirect("https://www.c-sharpcorner.com/");
}
RedirectToRouteResult
- Use in cases where route values require redirecting to an alternative action method inside the same application.
- especially when utilizing named routes, useful for navigating around the application.
- makes redirecting based on routing configuration possible in a more organized manner.
public ActionResult RedirectToAction()
{
return RedirectToAction("Index", "Home");
}
JsonResult
- Use in situations where you must give the client data in JSON format.
- Perfect for creating online APIs that use JSON for data interchange or for AJAX requests.
- makes it possible for client-side JavaScript frameworks to efficiently consume data without requiring refreshing entire pages.
public ActionResult GetJsonData()
{
var data = new { Name = "Peter", Age = 25 };
return Json(data, JsonRequestBehavior.AllowGet);
}
ContentResult
- Use this method when you have to send the client plain text or HTML as raw material.
- Ideal for producing dynamic content such as error warnings, delivering short replies, or presenting static material.
- allows you to create and deliver content directly from the controller action with more flexibility.
public ActionResult ContentText()
{
return Content("This is plain text content.");
}
FileResult
- Use this if you need to download a document or an image and then return it to the client.
- permits users to download data from the program, including Excel spreadsheets, photos, and PDFs.
- allows for the customization of the file name and content type while offering a smooth method of file serving.
public ActionResult DownloadFile()
{
byte[] fileBytes = System.IO.File.ReadAllBytes("path_to_file.pdf");
return File(fileBytes, "application/pdf", "filename.pdf");
}
HttpStatusCodeResult
- Use this when you need to provide the client with just a certain HTTP status number and no more content.
- useful for indicating different HTTP status codes, such as redirection, success, and client or server issues.
- permits appropriate HTTP status code processing to improve server-client communication.
public ActionResult Index()
{
// Return HTTP 404 (Not Found) status code
return new HttpStatusCodeResult(HttpStatusCode.NotFound);
}
EmptyResult
- Use in situations where an action method doesn't need to give the client any data; this is usually the case for actions that have side effects.
- Ideal for situations where the answer is not important in and of itself, like logging actions or updating database entries.
- helps in keeping the answer brief and effective when the client doesn't need any data to be returned.
​public ActionResult ClearCache()
{
// Code to clear cache goes here
// For example:
Cache.Clear();
// Return EmptyResult since no data needs to be sent back to the client
return new EmptyResult();
}
Developers have a lot of options when it comes to creating dynamic and responsive web apps with the diverse range of ActionResult types available in ASP.NET MVC. Through an understanding of the subtle differences between each ActionResult subtype and matching them to the particular needs of their applications, developers may coordinate smooth server-client interactions, improving the user experience in general.