It takes a thorough understanding of both backend data infrastructure and the newest features of C# 14 to enable CRUD operations in a GridView for report purposes when utilizing Amazon Redshift as the database of an ASP.NET MVC Core application. This article discusses in depth how this integration could be carried out in a robust, scalable manner with maximized performance and maintainability.
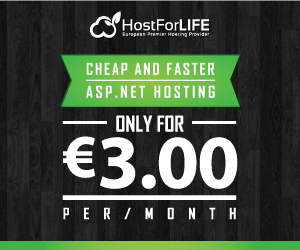
Setting Up the Environment
Prior to diving in with code, ensure your development environment contains.
ASP.NET Core MVC framework (most recent stable version)
C# 14 support is enabled in your project
AWS SDK for .NET and Redshift Data API Client
Entity Framework Core (EF Core) or Dapper for ORM (depending on your choice)
Connecting to Redshift
Redshift natively does not support direct connections using the usual EF Core due to its PostgreSQL heritage and some limitations. The secure, serverless way of accessing the Redshift Data API is what is recommended. The following NuGet package must be installed.
Install-Package AWSSDK.RedshiftDataAPIService
Establish a connection using your AWS credentials and set up the RedshiftDataClient.
var client = new AmazonRedshiftDataAPIServiceClient(
"your-access-key",
"your-secret-key",
RegionEndpoint.USEast1
);
CRUD Operations Implementation
Create
Let's insert records by invoking SQL commands through the Data API.
var sqlStatement = "INSERT INTO reports (title, content, created_at) VALUES (:title, :content, :created_at)";
var parameters = new List<SqlParameter>
{
new SqlParameter("title", report.Title),
new SqlParameter("content", report.Content),
new SqlParameter("created_at", DateTime.UtcNow)
};
await client.ExecuteStatementAsync(new ExecuteStatementRequest
{
Sql = sqlStatement,
Parameters = parameters,
ClusterIdentifier = "your-cluster-id",
Database = "your-database",
DbUser = "your-db-user"
});
Read
Let's fetch data for displaying in the GridView.
var response = await client.ExecuteStatementAsync(new ExecuteStatementRequest
{
Sql = "SELECT * FROM reports ORDER BY created_at DESC",
ClusterIdentifier = "your-cluster-id",
Database = "your-database",
DbUser = "your-db-user"
});
// Map the response to your model
Update
Let's update existing records.
var sqlStatement = "UPDATE reports SET title = :title, content = :content WHERE id = :id";
var parameters = new List<SqlParameter>
{
new SqlParameter("id", report.Id),
new SqlParameter("title", report.Title),
new SqlParameter("content", report.Content)
};
await client.ExecuteStatementAsync(new ExecuteStatementRequest
{
Sql = sqlStatement,
Parameters = parameters,
ClusterIdentifier = "your-cluster-id",
Database = "your-database",
DbUser = "your-db-user"
});
Delete
Let's remove records as follows.
var sqlStatement = "DELETE FROM reports WHERE id = :id";
var parameters = new List<SqlParameter>
{
new SqlParameter("id", report.Id)
};
await client.ExecuteStatementAsync(new ExecuteStatementRequest
{
Sql = sqlStatement,
Parameters = parameters,
ClusterIdentifier = "your-cluster-id",
Database = "your-database",
DbUser = "your-db-user"
});
Enrichments with C# 14 Features
C# 14 also features collection expressions and pattern matching enhancements that can make data mapping and data manipulation easier. For instance, parameter initialization list using collection expressions and result set processing using pattern matching can make your CRUD operations easier.
Security and Best Practices
- Call APIs securely using IAM Roles and Policies.
- Parameterize all SQLs to prevent SQL injection.
- Use aggressive error handling to catch exceptions nicely within a classically beautiful style.
- Max out Redshift's performance with sorts and named styles of distributions up to their capacities for doing them.
This trend is supported by a clean, green method of presenting report data in an ASP.NET Core MVC-based GridView with Amazon Redshift and C# 14-driven. Additional features can include asynchronous data loading with SignalR, dynamic filtering, and scheduling of reports.